Add Multiple Elements to a List in Python
In Python, to add multiple elements to a list, you can use methods like extend()
, append()
with iteration, and the +
operator.
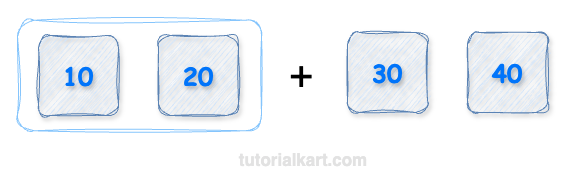
In this tutorial, we will explore various ways to add multiple elements to a list with examples.
Examples
1. Using the extend()
Method
The extend()
method allows adding multiple elements from an iterable (e.g., list, tuple, string) to an existing list.
# Creating an initial list
fruits = ["apple", "banana"]
# Adding multiple elements using extend()
fruits.extend(["cherry", "orange", "grape"])
# Printing the updated list
print("Updated List:", fruits)
Output:
Updated List: ['apple', 'banana', 'cherry', 'orange', 'grape']
The extend()
method adds each element from the iterable to the list individually.
2. Using the +
Operator
The +
operator can concatenate two lists, resulting in a new combined list.
# Creating an initial list
numbers = [1, 2, 3]
# Adding multiple elements using the + operator
new_numbers = numbers + [4, 5, 6]
# Printing the updated list
print("Updated List:", new_numbers)
Output:
Updated List: [1, 2, 3, 4, 5, 6]
Unlike extend()
, the +
operator creates a new list instead of modifying the original one.
3. Using append()
with a Loop
The append()
method adds a single element to a list at a time, but we can use a loop to add multiple elements.
# Creating an initial list
colors = ["red", "blue"]
# List of new colors to add
new_colors = ["green", "yellow", "purple"]
# Adding elements using a loop
for color in new_colors:
colors.append(color)
# Printing the updated list
print("Updated List:", colors)
Output:
Updated List: ['red', 'blue', 'green', 'yellow', 'purple']
Here, each element from new_colors
is added to the original list one at a time using a loop.
4. Using List Comprehension
List comprehension provides a concise way to add multiple elements to a list.
# Creating an initial list
letters = ["A", "B"]
# List of new elements to add
new_letters = ["C", "D", "E"]
# Adding elements using list comprehension
letters += [letter for letter in new_letters]
# Printing the updated list
print("Updated List:", letters)
Output:
Updated List: ['A', 'B', 'C', 'D', 'E']
This approach works similarly to extend()
but allows additional processing on elements if needed.
5. Using insert()
to Add Multiple Elements at a Specific Position
The insert()
method allows adding elements at a specific index. We can use a loop to insert multiple elements.
# Creating an initial list
animals = ["dog", "cat"]
# List of new elements to insert
new_animals = ["rabbit", "parrot"]
# Inserting elements at index 1
for animal in reversed(new_animals):
animals.insert(1, animal)
# Printing the updated list
print("Updated List:", animals)
Output:
Updated List: ['dog', 'rabbit', 'parrot', 'cat']
By using reversed(new_animals)
, we ensure that the elements maintain their original order when inserted.
Conclusion
Python provides multiple ways to add multiple elements to a list:
extend()
: Adds elements from an iterable to the existing list.+
Operator: Concatenates lists but creates a new one.append()
with a Loop: Adds elements one by one.- List Comprehension: Similar to
extend()
but more flexible. insert()
in a Loop: Adds elements at a specific position.
Choosing the right method depends on whether you want to modify the existing list or create a new one. The extend()
method is the most efficient way to add multiple elements to an existing list.