Create a List in Python
In Python, a list is a collection of items that can hold multiple values, including numbers, strings, or even other lists.
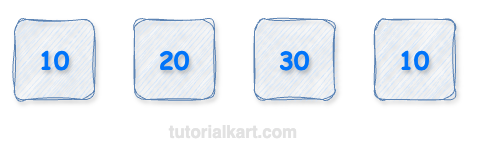
To create a List in Python, you can use square brackets []
or the list()
constructor. Let’s go through different ways to create a list in Python with examples.
Examples
1. Creating an Empty List in Python
We can create an empty list, which means the list will have no elements initially.
Here, we define an empty list using two different methods: by using empty square brackets []
and by using the list()
constructor.
# Creating an empty list using square brackets
empty_list1 = []
# Creating an empty list using list() constructor
empty_list2 = list()
# Printing both lists
print("First empty list:", empty_list1)
print("Second empty list:", empty_list2)
Output:
First empty list: []
Second empty list: []
Both methods create an empty list, which means they have no elements stored inside.
2. Creating a Python List with Elements
Lists can store multiple values, including numbers, strings, and even other lists.
To a create a list of items, you can use the following syntax:
my_list = [item1, item2, item3, ...]
In this example, we create three lists: one containing numbers, one containing strings, and one containing mixed data types.
# List of numbers
numbers = [1, 2, 3, 4, 5]
# List of strings
fruits = ["apple", "banana", "cherry"]
# List with mixed data types
mixed_list = [10, "Hello", 3.14, True]
# Printing the lists
print("Numbers List:", numbers)
print("Fruits List:", fruits)
print("Mixed List:", mixed_list)
Output:
Numbers List: [1, 2, 3, 4, 5]
Fruits List: ['apple', 'banana', 'cherry']
Mixed List: [10, 'Hello', 3.14, True]
Here, we created three different types of lists:
- A list of numbers where each item is an integer.
- A list of strings where each item is a word.
- A mixed list that contains different data types such as an integer, a string, a float, and a boolean.
3. Creating a List Using the list()
Constructor
We can also create a list using the list()
constructor.
Here, we use the list()
function to convert other iterable types such as strings, tuples, and ranges into a list.
# Creating a list from a string
char_list = list("hello")
# Creating a list from a tuple
tuple_list = list((1, 2, 3))
# Creating a list from a range
range_list = list(range(5))
# Printing the lists
print("List from string:", char_list)
print("List from tuple:", tuple_list)
print("List from range:", range_list)
Output:
List from string: ['h', 'e', 'l', 'l', 'o']
List from tuple: [1, 2, 3]
List from range: [0, 1, 2, 3, 4]
Here’s how each conversion works:
- When converting a string, each character becomes an element in the list.
- When converting a tuple, each element in the tuple becomes an element in the list.
- When converting a range, we get a list of numbers starting from 0 up to 4.
4. Creating a Nested List (List of Lists)
A list can also contain other lists as elements, forming a nested list.
Here, we create a nested list where each element is another list. This structure is useful when organizing data in rows and columns, like a table.
# Creating a nested list
nested_list = [[1, 2, 3], ["a", "b", "c"], [True, False, True]]
# Printing the nested list
print("Nested List:", nested_list)
# Accessing an inner list
print("Second inner list:", nested_list[1])
# Accessing an element inside a nested list
print("First element of second inner list:", nested_list[1][0])
Output:
Nested List: [[1, 2, 3], ['a', 'b', 'c'], [True, False, True]]
Second inner list: ['a', 'b', 'c']
First element of second inner list: a
Here:
- The main list contains three inner lists.
- We accessed the second inner list using
nested_list[1]
. - We accessed the first element of the second inner list using
nested_list[1][0]
.