Python assert
Keyword
The assert
keyword in Python is used for debugging purposes. It allows programmers to check if a condition is True
and raise an AssertionError
if it is not. This helps identify logical errors in the program during development.
Syntax
assert condition, optional_message
Parameters
Parameter | Type | Description |
---|---|---|
condition | Boolean expression | A condition that should evaluate to True . If False , an AssertionError is raised. |
optional_message | str, optional | An optional error message displayed if the assertion fails. |
Return Value
The assert
statement does not return anything. If the condition is True
, the program continues execution normally. If the condition is False
, an AssertionError
is raised, which stops the program unless handled.
Examples
1. Basic Assertion Example
In this example, we use an assert
statement to check if a number is positive.
We define a variable num
and use assert
to ensure that num
is greater than zero. If the condition is met, the program continues without interruption. If num
is zero or negative, an AssertionError
is raised.
# Define a number
num = 5
# Assert that num is positive
assert num > 0
# If assertion passes, this will be executed
print("The number is positive.")
Output:
The number is positive.
Since num
is 5, the assertion passes, and the program executes normally.
2. Assertion with a Custom Error Message
We can add an error message to make debugging easier.
Here, we assert that age
is greater than or equal to 18. If the condition fails, the message “Age must be 18 or above” is displayed along with the error.
# Define an age
age = 16
# Assert that age is at least 18
assert age >= 18, "Age must be 18 or above"
print("Access granted.")
Output:
Traceback (most recent call last):
File "/Users/tutorialkart/main.py", line 5, in <module>
assert age >= 18, "Age must be 18 or above"
^^^^^^^^^
AssertionError: Age must be 18 or above
Since age
is 16, which is less than 18, the assertion fails, and the error message is printed.
3. Using Assertions in a Function
Assertions are useful in functions to validate inputs.
Here, we define a function calculate_square_root
that computes the square root of a number. We use assert
to ensure the input is non-negative before performing the calculation.
import math
def calculate_square_root(num):
# Ensure the number is not negative
assert num >= 0, "Number must be non-negative"
return math.sqrt(num)
# Test with valid input
print(calculate_square_root(25))
# Test with invalid input
print(calculate_square_root(-4))
Output:
5.0
Traceback (most recent call last):
File "/Users/tutorialkart/main.py", line 13, in <module>
print(calculate_square_root(-4))
~~~~~~~~~~~~~~~~~~~~~^^^^
File "/Users/tutorialkart/main.py", line 5, in calculate_square_root
assert num >= 0, "Number must be non-negative"
^^^^^^^^
AssertionError: Number must be non-negative
When passing 25, the function returns 5.0. However, when passing -4, the assertion fails, raising an AssertionError
.
4. Disabling Assertions in Production Code
In Python, assertions can be disabled in optimized mode by running the script with the -O
flag.
Here, we define an assertion to check if value
is positive. However, when running Python in optimized mode, assertions are ignored, and the program executes normally.
# Define a value
value = -5
# Assert that value is positive
assert value > 0, "Value must be positive"
print("Program completed successfully.")
Running Normally:
Traceback (most recent call last):
File "/Users/tutorialkart/main.py", line 5, in <module>
assert value > 0, "Value must be positive"
^^^^^^^^^
AssertionError: Value must be positive
Running with -O
(Optimized Mode):
python -O script.py
Program completed successfully.
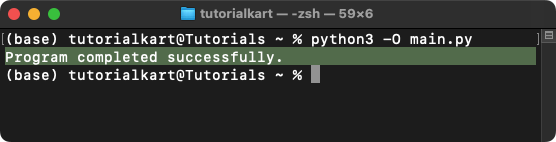
When running in optimized mode using python -O script.py
, the assertion is skipped, and no error is raised.