Python await
Keyword
The await
keyword in Python is used to pause the execution of an asynchronous function until the awaited result is available. It allows asynchronous functions to run efficiently without blocking the main thread.
Syntax
result = await coroutine_function()
How await
Works
The await
keyword can only be used inside an async
function. It tells Python to wait for an asynchronous operation to complete before continuing execution. This makes asynchronous programming more readable and efficient.
Return Value
The await
keyword returns the final result of the awaited coroutine when it completes execution.
Examples
1. Using await
with an Asynchronous Function
In this example, we define an async
function that simulates a time-consuming operation using asyncio.sleep()
. We then use await
to wait for it to complete.
import asyncio
# Define an asynchronous function
async def long_running_task():
print("Task started...")
await asyncio.sleep(2) # Simulate a delay
print("Task completed!")
return "Result from async function"
# Define another async function to call it
async def main():
print("Calling the async function...")
result = await long_running_task() # Wait for the async function to complete
print("Received result:", result)
# Run the event loop
asyncio.run(main())
Output:
Calling the async function...
Task started...
Task completed!
Received result: Result from async function
Explanation:
- The function
long_running_task()
starts execution but pauses atawait asyncio.sleep(2)
, simulating a delay. - During this time, Python can continue running other tasks.
- After 2 seconds, the function resumes execution and returns a result.
- The
main()
function awaits the result before continuing.
2. Running Multiple Asynchronous Tasks Concurrently
Instead of waiting for one task to complete before starting the next, we can run multiple tasks concurrently using asyncio.gather()
.
import asyncio
# Define an async function that simulates a task
async def async_task(name, delay):
print(f"Task {name} started...")
await asyncio.sleep(delay) # Simulate delay
print(f"Task {name} completed!")
return f"Result from {name}"
async def main():
# Run two tasks concurrently
task1 = async_task("A", 2)
task2 = async_task("B", 3)
results = await asyncio.gather(task1, task2) # Wait for both tasks to complete
print("All tasks completed with results:", results)
asyncio.run(main())
Output:
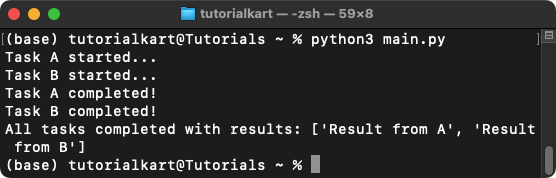
Explanation:
- Both tasks start simultaneously.
- Task A finishes after 2 seconds, while Task B finishes after 3 seconds.
- The
await asyncio.gather()
waits for both tasks to complete before printing the results.
3. Handling Errors in Asynchronous Functions
Errors can occur when awaiting an asynchronous function. Here, we demonstrate how to handle exceptions using try-except
.
import asyncio
# Define an async function that raises an error
async def faulty_task():
print("Task started...")
await asyncio.sleep(2)
raise ValueError("Something went wrong!")
async def main():
try:
await faulty_task()
except ValueError as e:
print("Caught an error:", e)
asyncio.run(main())
Output:
Task started...
Caught an error: Something went wrong!
Explanation:
- The function starts executing and pauses at
await asyncio.sleep(2)
. - After 2 seconds, it raises a
ValueError
. - The error is caught using a
try-except
block, preventing the program from crashing.
4. Using await
with an Asynchronous HTTP Request
We can use await
to perform non-blocking HTTP requests using the aiohttp
library.
import aiohttp
import asyncio
# Define an async function to fetch data
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text() # Await the response
async def main():
url = "https://www.example.com"
print("Fetching data...")
data = await fetch_data(url) # Await the result
print("Received data:", data[:100]) # Print first 100 characters
asyncio.run(main())
Explanation:
aiohttp
is used to perform an asynchronous HTTP GET request.await response.text()
ensures we don’t block execution while waiting for the response.- The function prints the first 100 characters of the fetched content.