In this Python GUI Tutorial, we will use Tkinter to learn how to develop GUI applications. We will learn how to get started with Tkinter, create some GUIs, and learn about the widgets in Tkinter.
Python GUI – Tkinter
To develop GUI application in Python, there are multiple options in terms of python packages. The most generally used package is tkinter.
While tkinter provides the widgets with the all the functionality and behavior aspects, there is another module named tkinter.ttk
which provides themed widget set.
Getting Started with Tkinter
Tkinter is an inbuilt python package. You can import the package and start using the package functions and classes.
import tkinter as tk
or you can use the other variation of importing the package
from tkinter import *
Create a Simple GUI Window
To create a GUI Window, tkinter provides Tk() class. The syntax of Tk() class is:
Tk(screenName=None, baseName=None, className=’Tk’, useTk=1)
Following is a simple example to create a GUI Window.
Python Program
import tkinter as tk
main_window = tk.Tk()
main_window.mainloop()
Output
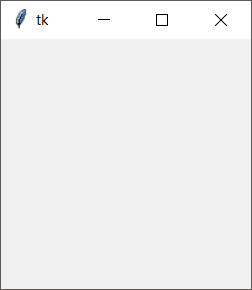
Python GUI Widgets
You can add widgets into the window. Also note that there are a wide variety of widgets you can use from tkinter. In this Tkinter Tutorial, we will cover all these widgets. Following are the list of Tkinter widgets.
- Button
- Canvas
- Checkbutton
- Radiobutton
- Entry
- Frame
- Label
- Listbox
- Menu
- MenuButton
- Message
- Scale
- Scrollbar
- Text
- TopLevel
- SpinBox
- PannedWindow
Tkinter Label Widget Tutorials
- How to set Color for Label Text in Tkinter Python?
- How to set Font Size for Label Text in Tkinter Python?
- How to set Italic Text for Label in Tkinter Python?
- How to set Bold Text for Label in Tkinter Python?
- How to set Font Family for Label in Tkinter Python?
- How to set Border for Label in Tkinter Python?
- How to set Background Color for Label in Tkinter Python?
- How to set Background Image for Label in Tkinter Python?
- How to display Text over an Image in Tkinter Python?
- How to Left Align Text in Label in Tkinter Python?
- How to Center Align Text in Label in Tkinter Python?
- How to Justify Text in Label in Tkinter Python?
- How to Right Align Text in Label in Tkinter Python?
- How to Change Text Color for Label on Hovering in Tkinter Python?
- How to Wrap Text in Label in Tkinter Python?
- How to Update Text in a Label on Button Click in Tkinter Python?
- How to Set Specific Width for Label in Tkinter Python?
- How to Set Specific Height for Label in Tkinter Python?
- How to Underline Text in Label in Tkinter Python?
- How to Strike-through Text in Label in Tkinter Python?
Tkinter Button Widget Tutorials
- Tkinter Button
- Tkinter Button Anchor
- How to Set Bold Text for Button in Tkinter Python
- How to Set Italic Text for Button in Tkinter Python
- How to set Button Active Background Color in Tkinter Python
- How to set Button Active Foreground Color in Tkinter Python
- How to set Button Background Color in Tkinter Python
- How to set Button Color in Tkinter Python
- How to Change Button Color after Clicking in Tkinter Python
- How to Change Button Color on Hovering in Tkinter Python
- How to set Button Border in Tkinter Python
- How to Set Button Border Color in Tkinter Python
- How to set Button command in Tkinter Python
- How to set Button Font in Tkinter Python
- How to set Button Foreground Color in Tkinter Python
- How to set Button Height in Tkinter Python
- How to set Button Width in Tkinter Python
- How to Set the Size of Button in Tkinter Python
- How to Disable the Button After Click in Tkinter Python
- How to Call a Function on Button Click in Tkinter Python
- How to Call a Function with Arguments on Button Click in Tkinter Python
Tkinter Entry Widget Tutorials
- How to get Text Entered in Entry Widget in Tkinter Python
- How to set a Value in Entry Widget in Tkinter Python
- How to Focus Entry Widget in Tkinter Python
- How to set Width for Entry Widget in Tkinter Python
- How to set Placeholder for Entry Widget in Tkinter Python
- How to use Entry Widget for Password in Tkinter Python
- How to Toggle Password Visibility in Entry Widget in Tkinter Python
- How to setup Validation for Entry Widget in Tkinter Python
- How to Limit Characters in Entry Widget in Tkinter Python
- How to set a Default Value for Entry Widget in Tkinter Python
- How to Auto-resize Entry Widget in Tkinter Python
- How to Auto-resize Entry Widget based on Input in Tkinter Python
- How to set Background Color for Entry Widget in Tkinter Python
- How to set Font Color for Entry Widget in Tkinter Python
- How to set Font Size for Entry Widget in Tkinter Python
- How to set Bold Font for Entry Widget in Tkinter Python
- How to Center Align Text in Entry Widget in Tkinter Python
- How to setup Change Event for Entry Widget in Tkinter Python
- How to clear Entry Widget on Button Click in Tkinter Python
- How to disable Entry Widget on Button Click in Tkinter Python
- How to Delete Last Character in Entry Widget in Tkinter Python
- What are the Events available for Entry Widget in Tkinter Python
- How to get Cursor Position in Entry Widget in Tkinter Python
- How to Allow Only Integers in Entry Widget in Tkinter Python
- How to Right Align Text in Entry Widget in Tkinter Python
- How to Change Background Color of Entry Widget on Hover in Tkinter Python
- How to Change Background Color of Entry Widget on Focus in Tkinter Python
- How to Implement Undo and Redo for Entry Widget in Tkinter Python
Tkinter Text Widget Tutorials
- How to get Value Entered in Text Widget in Tkinter Python
- How to set a Value in Text Widget in Tkinter Python
- How to Focus Text Widget in Tkinter Python
- How to set Specific Width for Text Widget in Tkinter Python
- How to set Specific Height for Text Widget in Tkinter Python
- How to set Specific Size for Text Widget in Tkinter Python
- How to set Placeholder for Text Widget in Tkinter Python
- How to set Auto-scroll for Text Widget in Tkinter Python
- How to set Horizontal Scrollbar for Text Widget in Tkinter Python
- How to set Vertical Scrollbar for Text Widget in Tkinter Python
- How to setup Validation for Text Widget in Tkinter Python
- How to Limit Characters in Text Widget in Tkinter Python
- How to set a Default Value for Text Widget in Tkinter Python
- How to Wrap Content in Text Widget in Tkinter Python
- How to Auto-resize Text Widget based on Input in Tkinter Python
- How to set Background Color for Text Widget in Tkinter Python
- How to set Font Color for Text Widget in Tkinter Python
- How to Set Different Font Colors for Different Parts of Text Widget in Tkinter Python
- How to set Font Size for Text Widget in Tkinter Python
- How to set Font Family for Text Widget in Tkinter Python
- How to set Bold Font for Text Widget in Tkinter Python
- How to Center Align Text in Text Widget in Tkinter Python
- How to call a Function when the Text Changes in a Text Widget in Tkinter Python
- How to clear Text Widget on Button Click in Tkinter Python
- How to disable Text Widget on Button Click in Tkinter Python
- How to disable Text Widget with a Default Value in it in Tkinter Python
- How to Delete Last Character in Text Widget in Tkinter Python
- What are the Different Events available for Text Widget in Tkinter Python
- How to get Cursor Position in Text Widget in Tkinter Python
- How to Allow Only Numbers in Text Widget in Tkinter Python
- How to Right Align Text in Text Widget in Tkinter Python
- How to Change Background Color of Text Widget on Hover in Tkinter Python
- How to Change Background Color of Text Widget on Focus in Tkinter Python
Tkinter Issues – Solved
While working with Tkinter, you may come across some of the following issues.
- Python Tkinter Frame Width Height Not Working
- Python ModuleNotFoundError: No module named ‘_tkinter’ on Mac
Conclusion
In this Python Tutorial, we learned about Tkinter library and the widgets it provides to build a GUI application in Python.