Functions in R
We shall learn about types of R functions, function’s syntax and how to call a defined function from other part of the R script file.
What is a function in R?
A function is a set of instructions to perform a specific task. In a programming language, a function transforms the arguments provided into a desired form.
For example, consider a function that does addition. It calculates the sum of provided arguments. The calculation part is the transformation and final sum is the desired form of arguments.
R programming language provides functions to group a set of instructions and form a task.There are two types of functions in R language. They are :
- Built-in R functions
- User defined R functions
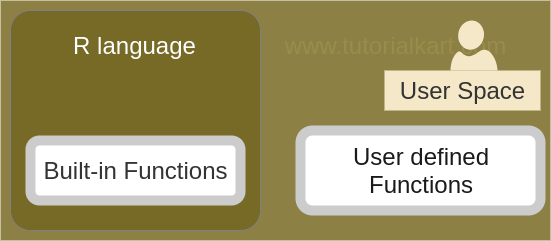
Built-in R function
Any programming language has been built based on a requirement and the development of it progresses with its vision. As R programming language has been built for data analytics, most of the commonly used analytical tasks are provided by R as built-in functions. So that these tasks could be accessible to a developer and to avoid rewriting of the trivial functions.
Following are some of the built-in R functions provided :
cos(x), sin(x), tan(x), sum(range), mean(range) etc.
User defined R functions
Some scenarios demand you to write functions whether it is to implement a new algorithm or write your business logic. For such cases, R programming language provides ability to write user defined functions.
Following is an example of user defined R function, where you need to implement an addition of three numbers.
# R function
addition = function(a,b){
return (a+b)
}
Syntax – R function
The syntax of an R function is
function_name = function(arguments){
function_body
}
function_name – Required : name of the function by which you want to reference it from rest of the code
arguments – Optional : the values that are going to be used inside the function block.
function_body – Optional : the set of statements which perform a task collectively. Body of the function may contain return_value. return_value is the final result of transformation of arguments.
Calling an R Function
Once a function is defined, you may call it from other part of R script file. We shall learn with example R scripts to call a function in the following scenarios :
- r function that has no arguments
- r function that has arguments
- r function that returns a value
Example 1 – R function that has no arguments
In this example, we will write a function that takes no arguments.
r_function_no_args.R
# R function with no arguments
sayHello = function(){
print("Hello !")
}
sayHello()
Output
$ Rscript r_function_no_args.R
[1] "Hello !"
The function has no arguments.
Example 2 – R function that has arguments
In this example, we will write a function with three parameters. So, when we are calling this function, we have to pass three values as arguments.
r_function_args.R
# R function with arguments
addition = function(a,b,c){
print(a+b+c)
}
addition(4,15,6)
Output
$ Rscript r_function_args.R
[1] 25
The function accepts arguments, does addition and prints the result to console.
Example 3 – R function that returns a value
In this example, we will write a function that returns a value. We will store this returned value in a variable, say d
.
r_function_return.R
# R function with arguments and return value
addition = function(a,b,c){
return (a+b+c)
}
d = addition(4,15,6)
print(d)
Output
$ Rscript r_function_return.R
[1] 25
The function accepts arguments, does addition and returns the result to the point of function calling.
Conclusion
In this R Tutorial, we have learnt about types of R functions, their syntax and how to call a defined function from other part of the R script file.