Swift – Create Directory at Specific Path
To create a Directory at specific path in Swift, call createDirectory()
function on the file manager object.
The following code snippet creates Directory at path “/ab/cd/”.
FileManager.default.createDirectory(atPath: "/ab/cd/", withIntermediateDirectories: true, attributes: nil)
withIntermediateDirectories: true
creates intermediate directories if not present. We can also provide optional file attributes via attributes
parameter.
Example
In the following example Swift program, we will create a directory at path "/Users/tutorialkart/test1/"
.
main.swift
import Foundation
let dirPath = "/Users/tutorialkart/test1/"
do {
try FileManager.default.createDirectory(atPath: dirPath, withIntermediateDirectories: true, attributes: nil)
} catch {
print(error)
}
Output
A directory with the specified path is successfully created.
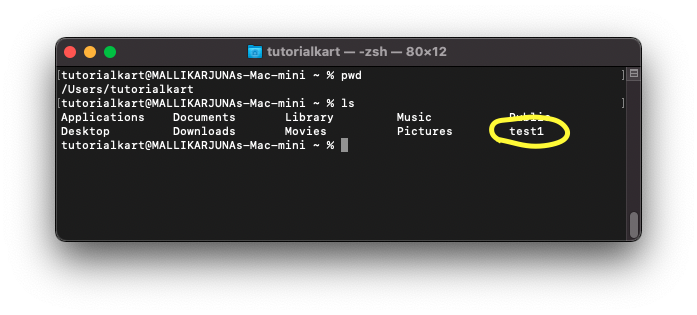
Now, let us create another directory, where intermediate directories are not present. We shall pass true
for withIntermediateDirectories
parameter, therefore, intermediate directories should be created without difficulty.
main.swift
import Foundation
let dirPath = "/Users/tutorialkart/test2/test3/test4/"
do {
try FileManager.default.createDirectory(atPath: dirPath, withIntermediateDirectories: true, attributes: nil)
} catch {
print(error)
}
Output
A directory with the specified path is successfully created.
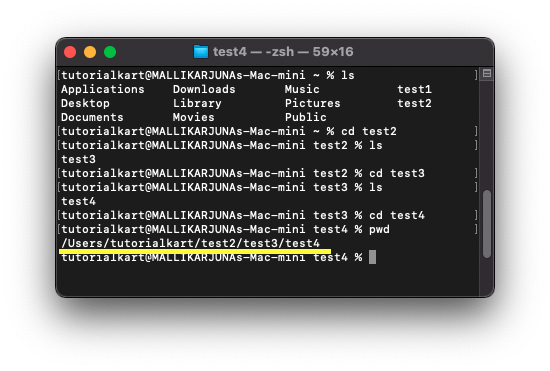
Conclusion
In this Swift Tutorial, we learned how to create a directory at specific path, with examples.