Swift Division Operator
To perform division of two numbers in Swift, we can use Swift Division Arithmetic operator.
Division Operator /
takes two numbers as operands and returns the quotient when the first operand is divided by the second operand.
If the two operands are integers, then division operator returns the quotient, which could leave a reminder.
If the two operands are Float or Double, then division operator returns the quotient to the best possible point precision.
Syntax
The syntax to find the division of two numbers is
a / b
where a
and b
are operands.
These operands should be of same type. For example
a
andb
can be Int.a
andb
can be Float.a
andb
can be Double.
Examples
1. Division with integer values
In the following program, we will perform division of two integers.
main.swift
var a = 11 var b = 4 var result = a / b print("The result is \(result)")
Output
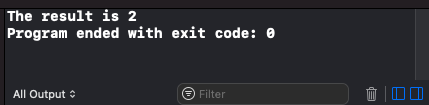
2. Division with Double values
Now, let us find the division of two Double values.
main.swift
var a = 11.5 var b = 4.2 var result = a / b print("The result is \(result)")
Output
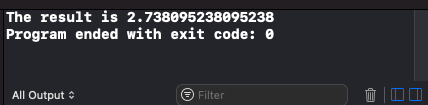
3. Division with values of different datatypes
If we try division with numbers of different types, we would get Swift Compiler Error as shown below.
main.swift
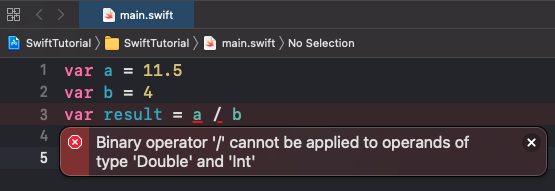
Since a
is Double and b
is Int, Division -
operator raises Compiler Error.
Conclusion
In this Swift Tutorial, we learned how to perform Division in Swift programming using Division Operator. We have seen the syntax and usage of Division operator, with examples.