Swift String Array – Check if Specific String is Present
To check if a specific string is present in a String array in Swift, call contains(_:)
method on the String Array and pass the specific String as argument to the contains() method.
Syntax
The syntax to call contains() method to check if the specific string str
is present in String Array strArray
is
strArray.contains(str)
Examples
In the following program, we will take a string array and check if the string "mango"
is present in this array.
main.swift
var strArr = ["apple", "banana", "cherry", "mango", "guava"] var str = "mango" var isStrPresent = strArr.contains(str) print("Is string present in array? \(isStrPresent)")
Output
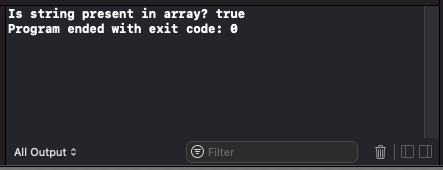
Since str
is present in strArr
, contains() method returned true
.
Now, let us take the string array and string we would like to search in such a way that the string is not present in the string array.
main.swift
var strArr = ["apple", "banana", "cherry", "mango", "guava"] var str = "avacado" var isStrPresent = strArr.contains(str) print("Is string present in array? \(isStrPresent)")
Output
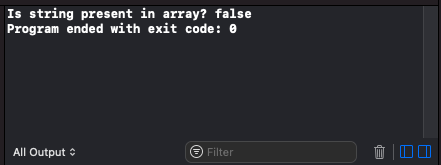
Since str
is not present in strArr
, contains() method returned false
.
Conclusion
In this Swift Tutorial, we learned how to check if a String Array contains a Specific String in Swift programming.