Arithmetic Overflow Operators in Swift
In Swift, arithmetic overflow operators are used to handle situations where a calculation exceeds the range of the data type. By default, Swift prevents overflow and throws a runtime error for invalid operations. However, overflow operators allow calculations to continue by discarding overflowed bits, resulting in a “wrapped around” value.
Arithmetic Overflow Operators in Swift
Here are the overflow operators provided by Swift:
Operator | Description | Example |
---|---|---|
&+ | Overflow addition | Wraps around on overflow |
&- | Overflow subtraction | Wraps around on overflow |
&* | Overflow multiplication | Wraps around on overflow |
Example: Overflow Addition
This example demonstrates the use of the &+
operator for overflow addition:
File: main.swift
let maxValue = UInt8.max
print("Max Value: \(maxValue)")
// Overflow addition
let result = maxValue &+ 1
print("Result after overflow addition: \(result)")
Explanation:
UInt8.max
represents the maximum value of an 8-bit unsigned integer, which is 255.- The
&+
operator performs addition but allows the value to wrap around upon overflow. - Adding 1 to 255 results in 0 due to wrapping around.
Output:
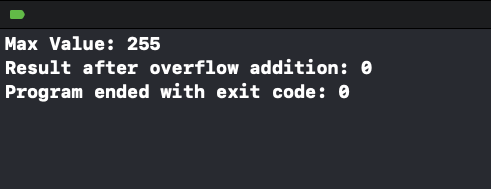
Example 1: Overflow Subtraction
This example demonstrates the use of the &-
operator for overflow subtraction:
File: main.swift
let minValue = UInt8.min
print("Min Value: \(minValue)")
// Overflow subtraction
let result = minValue &- 1
print("Result after overflow subtraction: \(result)")
Explanation:
UInt8.min
represents the minimum value of an 8-bit unsigned integer, which is 0.- The
&-
operator performs subtraction but allows the value to wrap around upon underflow. - Subtracting 1 from 0 results in 255 due to wrapping around.
Output:
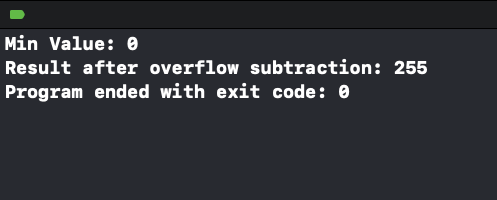
Example 2: Overflow Multiplication
This example demonstrates the use of the &*
operator for overflow multiplication:
File: main.swift
let value: UInt8 = 128
// Overflow multiplication
let result = value &* 2
print("Result after overflow multiplication: \(result)")
Explanation:
- The value
128
is multiplied by 2 using the&*
operator. - The result exceeds the maximum value for
UInt8
(255) and wraps around to produce the value0
.
Output:
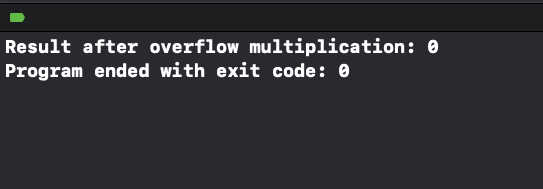
Use Cases for Arithmetic Overflow Operators
Arithmetic overflow operators are particularly useful in scenarios where performance is critical, such as:
- Low-level programming: Working with hardware or binary data that relies on wrapping behavior.
- Graphics programming: Performing calculations that require precise overflow handling.
- Cryptography: Implementing algorithms where overflow behavior is expected and necessary.
Conclusion
Swift’s arithmetic overflow operators provide a safe and efficient way to handle overflow and underflow scenarios in calculations.