Swift Array – ForEach
To iterate over each element of a Swift Array, we can use forEach and access each element during respective iteration.
The following code snippet demonstrates how to use forEach statement with array, and iterate over each element of the array.
array.forEach { element in
//code
}
where array
is the array of elements, and element
contains that element of the array during that iteration.
Note: Please note that we cannot use break or continue statements inside forEach statement. Therefore forEach statement executes the forEach block for each of the element in array.
Examples
In the following program, we will take a string array, fruits
, and iterate over each of the element using forEach statement.
main.swift
var fruits:[String] = ["Apple", "Banana", "Mango"]
fruits.forEach { fruit in
print(fruit)
}
Output
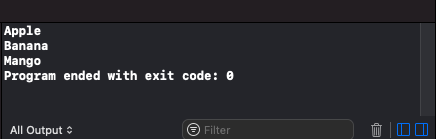
Now, let us take an array of integers, and iterate over this array using forEach statement.
main.swift
var primes:[Int] = [2, 3, 5, 7, 11]
primes.forEach { prime in
print(prime)
}
Output
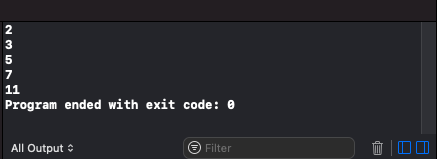
Conclusion
Concluding this Swift Tutorial, we learned how to iterate over array elements using forEach statement in Swift.