Swift Array – Remove First Element
To remove first element from Array in Swift, call remove(at:)
method on this array and pass the index 0
for at
parameter. Or call removeFirst() method on the array.
remove(at:)
method removes the element at given index.
The syntax to call remove(at:)
method on the array with index
is
arrayName.remove(at:index)
In this particular scenario, where we would like to remove the first element, the value of index
we pass is 0
.
The syntax to call removeFirst()
method on the array is
arrayName.removeFirst()
removeFirst() method removes and returns the first element of the array.
Examples
In the following program, we will take an array, and remove the first element using remove(at:) method.
main.swift
var fruits = ["apple", "banana", "cherry", "mango", "guava"]
fruits.remove(at:0)
print(fruits)
Output
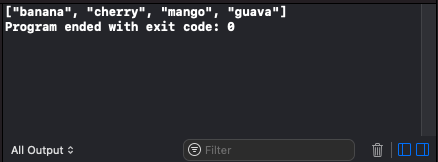
The first element "apple"
has been removed from the array fruits
.
If the array is empty, removing first element using remove(at:)
causes a runtime error as shown in the following example.
main.swift
var fruits: [String] = []
fruits.remove(at:0)
print(fruits)
Output
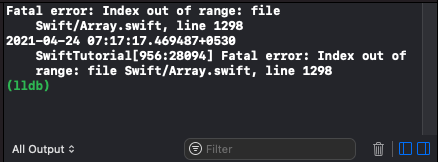
Remove First Element using Array.removeFirst()
In the following example, we will use removeFirst() method and remove the first element in the array.
main.swift
var fruits = ["apple", "banana", "cherry", "mango", "guava"]
fruits.removeFirst()
print(fruits)
Output
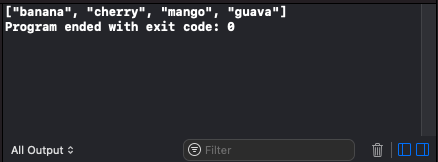
Conclusion
In this Swift Tutorial, we learned how to remove first element from array in Swift.