Swift Array Reverse
To reverse a Swift Array, call reverse() or reversed() method on the array.
reverse() method reverses the elements of the array in place.
reversed() method creates a new array with the elements of the original array reversed, and returns this new array.
Examples
In this tutorial, we will go through examples covering reverse() and reversed() methods.
reverse()
In the following program, we will take an array, and reverse the order of its elements using reverse() method.
main.swift
</>
Copy
var arr = [1, 2, 3, 8, 25, 99]
arr.reverse()
print(arr)
Output
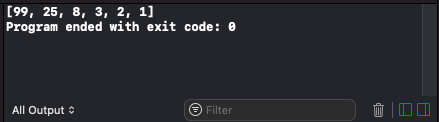
reversed()
In the following program, we will take an array, and reverse the order of its elements using reversed() method.
main.swift
</>
Copy
var arr = [1, 2, 3, 8, 25, 99]
var arrReversed: [Int] = arr.reversed()
print("Original Array: \(arr)")
print("Reversed Array: \(arrReversed)")
Output
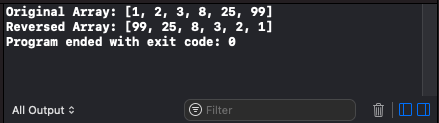
Conclusion
In this Swift Tutorial, we learned how to reverse a Swift Array using reverse() or reversed() method.