Swift Array Sorting
To sort an Array in Swift, we can call any of the method on the array (mentioned in the following table), based on the sorting behaviour, and inplace sorting.
In this tutorial, we will go through each of these methods with examples.
Method | Inplace Sorting | Condition to Sort |
---|---|---|
sort() | Yes | Implicit sorting condition |
sort(by:) | Yes | Explicit sorting condition provided via by parameter |
sorted() | No | Implicit sorting condition |
sorted(by:) | No | Explicit sorting condition provided via by parameter |
sort()
sort() method sorts the array in place, meaning the original array is modified.
Example
In the following program, we will take an array of numbers and sort them using sort() method.
main.swift
var arr = [2, 8, 1, 9, 4, 7]
arr.sort()
print(arr)
Output
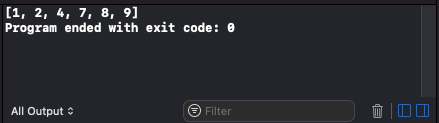
sort(by:)
sort(by:) method sorts the array in place using the given predicate as the comparison between elements. In place sorting means, the original array is modified.
Example
In the following program, we will take an array of strings and sort them using sort(by:) method, based on the condition that the string length decides which string is greater.
main.swift
var ids = ["ab", "qprs", "mno", "vwxyz", "c"]
ids.sort(by: {$0.count < $1.count})
print(ids)
Output
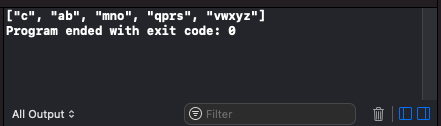
sorted()
sorted() method sorts a new array with the elements of original array sorted. Contents of original array remain unchanged.
Example
In the following program, we will take an array of numbers and sort them using sorted() method.
main.swift
var ids = [2, 8, 1, 9, 4, 7]
var sortedIds = ids.sorted()
print("Original Array: \(ids)")
print("Sorted Array: \(sortedIds)")
Output
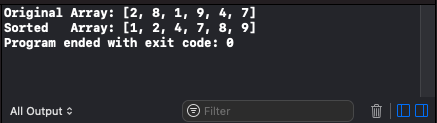
sorted(by:)
sorted(by:) method creates a new array with the elements of the original array sorted using the given predicate as the comparison between elements. Original array remain unchanged.
Example
In the following program, we will take an array of strings and sort them using sorted(by:) method, based on the condition that the string length decides which string is greater.
main.swift
var strArr = ["ab", "qprs", "mno", "vwxyz", "c"]
var sortedArr = strArr.sorted(by: {$0.count < $1.count})
print("Original Array: \(strArr)")
print("Sorted Array: \(sortedArr)")
Output
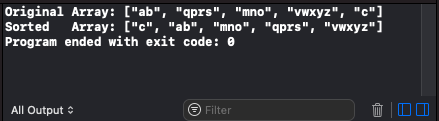
Conclusion
In this Swift Tutorial, we learned how to sort an Array in Swift using different methods like sort(), sort(by:), sorted() and sort(by:).