Swift Bitwise Operators
In Swift, bitwise operators are used to manipulate individual bits within integers. These operators are essential for tasks such as low-level programming, optimizing performance, and working with binary data. Bitwise operations allow you to perform AND, OR, XOR, and other operations directly on the binary representation of numbers.
Bitwise Operators in Swift
Here is a list of bitwise operators available in Swift:
Operator | Description | Example |
---|---|---|
& | Bitwise AND | Sets each bit to 1 if both bits are 1 |
| | Bitwise OR | Sets each bit to 1 if one of the bits is 1 |
^ | Bitwise XOR | Sets each bit to 1 if only one of the bits is 1 |
~ | Bitwise NOT | Inverts all the bits |
<< | Left Shift | Shifts bits to the left by a specified number of positions |
>> | Right Shift | Shifts bits to the right by a specified number of positions |
Example: Using Bitwise Operators
This example demonstrates the basic use of bitwise operators:
File: main.swift
let a: UInt8 = 0b10101010
let b: UInt8 = 0b11001100
// Bitwise AND
let andResult = a & b
print("AND result: \(String(andResult, radix: 2))")
// Bitwise OR
let orResult = a | b
print("OR result: \(String(orResult, radix: 2))")
// Bitwise XOR
let xorResult = a ^ b
print("XOR result: \(String(xorResult, radix: 2))")
// Bitwise NOT
let notResult = ~a
print("NOT result: \(String(notResult, radix: 2))")
Explanation:
a
andb
are binary numbers represented in an 8-bit format.- The
&
operator performs a bitwise AND operation, keeping bits that are 1 in botha
andb
. - The
|
operator performs a bitwise OR operation, keeping bits that are 1 in eithera
orb
. - The
^
operator performs a bitwise XOR operation, keeping bits that are 1 in eithera
orb
but not both. - The
~
operator inverts all bits ina
.
Output:
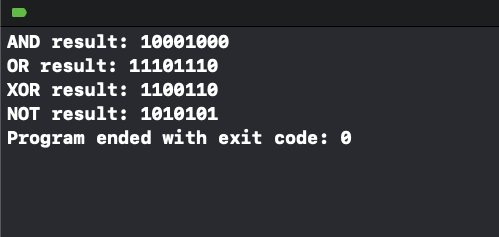
Example 1: Left and Right Shift
This example demonstrates bitwise shifting: both left and right. We take an unsigned integer with eight bits, and shift if left by 2 bits, and the shift the same value right by 2 bits, and print the outputs.
File: main.swift
let value: UInt8 = 0b00001111
// Left shift
let leftShift = value << 2
print("Left shift result: \(String(leftShift, radix: 2))")
// Right shift
let rightShift = value >> 2
print("Right shift result: \(String(rightShift, radix: 2))")
Explanation:
- The
<<
operator shifts the bits ofvalue
to the left by 2 positions, adding zeros on the right. - The
>>
operator shifts the bits ofvalue
to the right by 2 positions, discarding bits on the right.
Output:
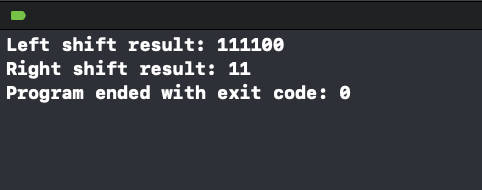
Example 2: Masking and Filtering Bits using Bitwise Operators
This example shows how to use bitwise operators for masking:
File: main.swift
let value: UInt8 = 0b11010101
let mask: UInt8 = 0b00001111
// Apply mask
let maskedValue = value & mask
print("Masked result: \(String(maskedValue, radix: 2))")
Explanation:
- The mask
0b00001111
isolates the last 4 bits of thevalue
. - The
&
operator ensures only the last 4 bits ofvalue
remain.
Output:
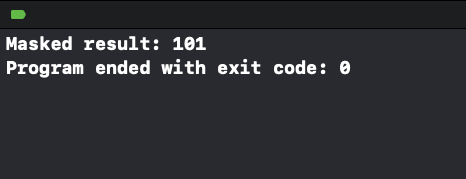
Conclusion
Bitwise operators are powerful tools for working directly with binary data in Swift. They are commonly used in performance-critical applications, graphics programming, and low-level hardware interactions.