Swift Boolean
Booleans are one of the most fundamental data types in Swift. They represent logical values: true
or false
. Boolean values are essential for controlling program flow through conditional statements and loops.
In this tutorial, you will learn about boolean data type in Swift, how to use them in conditional statements and loops, with examples.
Boolean Data Type
In Swift, the Boolean type is Bool
. It can hold one of two values:
true
: Represents a logically true condition.false
: Represents a logically false condition.
Here’s an example of declaring Boolean variables:
File: main.swift
let isSwiftFun: Bool = true
let isProgrammingHard: Bool = false
print("Is Swift fun? \(isSwiftFun)")
print("Is programming hard? \(isProgrammingHard)")
Output:
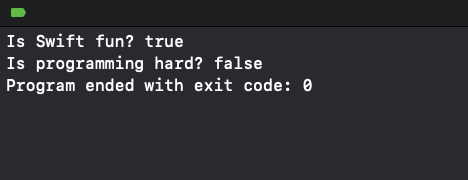
Using Booleans in Conditional Statements
Boolean values are commonly used in conditional statements to determine the flow of execution:
File: main.swift
let hasCompletedTask = true
if hasCompletedTask {
print("Great job! You've completed the task.")
} else {
print("Keep going! You're almost there.")
}
Explanation:
- The
if
statement checks the value ofhasCompletedTask
. - If the value is
true
, the first block of code is executed; otherwise, theelse
block is executed.
Output:
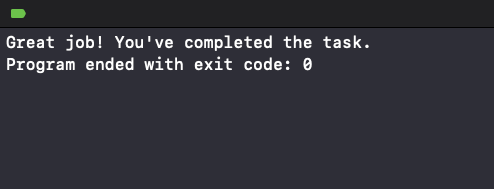
Combining Booleans with Logical Operators
Swift provides logical operators to combine or negate Boolean values:
&&
: Logical AND||
: Logical OR!
: Logical NOT
File: main.swift
let isRaining = false
let hasUmbrella = true
if !isRaining || hasUmbrella {
print("You can go outside.")
} else {
print("Stay indoors to avoid getting wet.")
}
Explanation:
- The
!isRaining
negates the value ofisRaining
, making ittrue
. - The
||
operator checks if at least one of the conditions (!isRaining
orhasUmbrella
) is true. - If either condition is true, the program executes the first block of code.
Output:
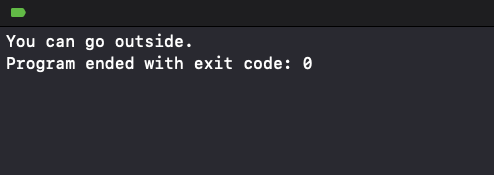
Booleans in Loops
Booleans are also used in loops to control repetition:
File: main.swift
var isRunning = true
var counter = 0
while isRunning {
print("Counter: \(counter)")
counter += 1
if counter == 5 {
isRunning = false
}
}
Explanation:
- The
while
loop continues as long asisRunning
istrue
. - Inside the loop,
counter
is incremented, and the loop terminates whencounter
reaches 5.
Output:
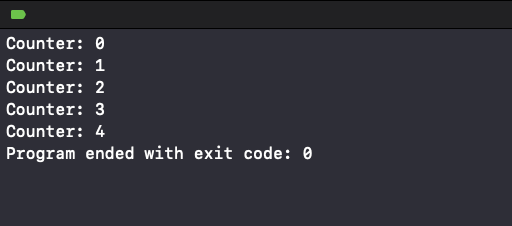
Conclusion
The Boolean type is an essential part of Swift programming, enabling logic and control flow in your applications.