Swift Characters
Characters are the building blocks of strings in Swift. A Character represents a single Unicode character, allowing for the representation of letters, digits, symbols, and other special characters. Swift’s support for Unicode ensures that it can handle characters from many languages and symbols from various writing systems.
Declaring a Character in Swift
Characters in Swift are represented by the Character
type. Here’s an example:
File: main.swift
let letter: Character = "A"
let digit: Character = "1"
let symbol: Character = "@"
print("Letter: \(letter)")
print("Digit: \(digit)")
print("Symbol: \(symbol)")
Explanation:
letter
: Represents the characterA
.digit
: Represents the character1
.symbol
: Represents the character@
.
Output:
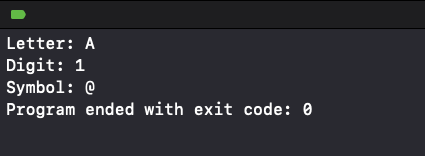
Iterating Over Characters in a String
You can access each character in a string using a loop:
File: main.swift
let greeting = "Hello"
for character in greeting {
print(character)
}
Explanation:
- The
for
loop iterates over eachCharacter
in the stringgreeting
. - Each character is printed on a new line.
Output:
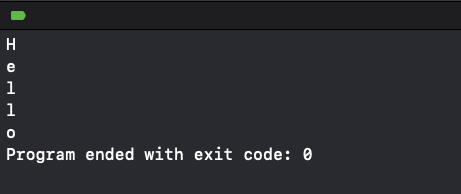
Combining Characters into a String
Characters can be combined to form a string:
File: main.swift
let char1: Character = "S"
let char2: Character = "w"
let char3: Character = "i"
let char4: Character = "f"
let char5: Character = "t"
let word = String([char1, char2, char3, char4, char5])
print("Word: \(word)")
Explanation:
- Characters are stored in an array and converted to a string using the
String
initializer. - The resulting string
"Swift"
is printed.
Output:
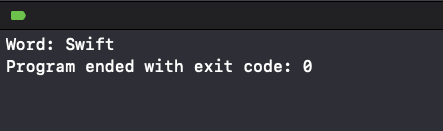
Special Unicode Characters
Swift supports special Unicode characters such as emojis:
File: main.swift
let heart: Character = "❤️"
let smiley: Character = "😊"
print("Heart: \(heart)")
print("Smiley: \(smiley)")
Explanation:
- Unicode characters like emojis are stored as
Character
values. - They are printed just like regular characters.
Output:
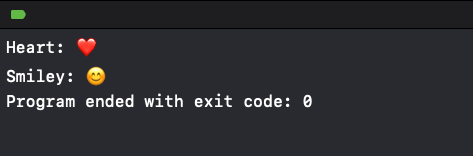
Comparing Characters
Characters can be compared using relational operators:
File: main.swift
let char1: Character = "A"
let char2: Character = "B"
if char1 < char2 {
print("\(char1) comes before \(char2)")
} else {
print("\(char1) does not come before \(char2)")
}
Explanation:
- Characters are compared based on their Unicode values.
- The program determines whether
char1
comes beforechar2
.
Output:
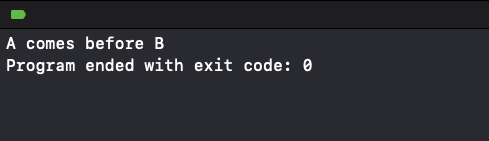
Conclusion
Understanding the Character
type in Swift is essential for working with strings and Unicode data. Characters are versatile and can represent a wide range of symbols and letters.