In this tutorial, you will learn how to check if given path is a directory or not in Swift language using fileExists() method.
Check if given Path is a Directory in Swift
To check if given path is a directory or not, in Swift, follow these steps.
- Given a path.
- Get the default shared File manager using FileManager.defaut.
- Take a boolean variable of type ObjCBool, and initialise it to false, say isDir.
- Call fileExists() method of the file manager, and pass the path and boolean variable isDir as arguments.
- If the given path is a directory, then the given boolean value isDir is set to true.
- Or if the given path is not a directory, then the given boolean value isDir is set to false.
- You can use a Swift if-else statement with the boolean value isDir as if-condition.
The syntax to call fileExists() method with path and boolean variable passed as arguments is
fileExists(atPath: path, isDirectory: &isDir)
Examples
1. Check if given path is a directory
In this example, we take a path "/Users/tutorialkart/"
, and check if this path is a directory or not.
main.swift
import Foundation
let path = "/Users/tutorialkart/"
let fileManager = FileManager.default
var isDir: ObjCBool = false
fileManager.fileExists(atPath: path, isDirectory: &isDir)
if isDir.boolValue {
print("Given path is a DIRECTORY.")
} else {
print("Given path is NOT a DIRECTORY.")
}
Output
We have taken the path such that the path is a directory. Therefore, isDir variable is set to true, and the if-block is executed.
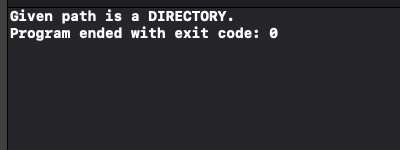
2. Given path is not a directory
In this example, we take a path "/Users/tutorialkart/data.txt"
, and check if this path is a directory.
main.swift
import Foundation
let path = "/Users/tutorialkart/data.txt"
let fileManager = FileManager.default
var isDir: ObjCBool = false
fileManager.fileExists(atPath: path, isDirectory: &isDir)
if isDir.boolValue {
print("Given path is a DIRECTORY.")
} else {
print("Given path is NOT a DIRECTORY.")
}
Output
The given path is a text file, and is not a directory. Therefore, isDir is set to false, and else-block is executed.
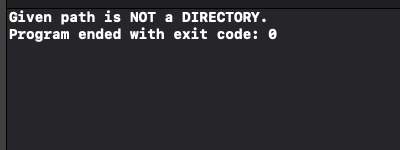
Conclusion
In this Swift Tutorial, we have seen how to check if a given path is a directory or not, using fileExists() method, with examples.