Swift Classes
Classes are one of the fundamental building blocks of object-oriented programming in Swift. A class defines a blueprint for creating objects that encapsulate properties (data) and methods (functions) into a single entity.
Classes in Swift also support features such as inheritance, reference semantics, and initialisers, making them a powerful tool for modelling real-world entities and relationships in code.
Declaring a Class
Declaring a class in Swift requires the class
keyword followed by the class name. The name should start with an uppercase letter by convention. The body of the class is enclosed in curly braces {}
, where you define the class’s properties and methods.
Syntax:
class ClassName {
// Properties
// Methods
}
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func displayDetails() {
print("Name: \(name), Age: \(age)")
}
}
Explanation:
class
: The keyword used to declare a class.Student
: The name of the class, starting with an uppercase letter.- Curly braces: Enclose the class’s properties and methods.
name
andage
: Properties to store the student’s name and age.init
: An initializer used to set up properties when a new object is created.displayDetails
: A method to print the student’s details.
Properties
Properties in a class are variables or constants that store data. They are declared inside the class body and are typically initialized either directly or through an initializer.
Example: main.swift
class Student {
var name: String
var age: Int
}
Properties can be accessed and modified using dot notation, as shown later in this tutorial.
Methods
Methods are functions that are defined inside a class and operate on its properties. They can perform actions or return values based on the class’s properties.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func greet() {
print("Hello, my name is \(name).")
}
}
Explanation:
greet
: A method that prints a greeting message using thename
property.
Initializers
An initializer is a special method in a class that sets up the initial state of an object by assigning values to its properties. It is defined using the init
keyword.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
Explanation:
init
: The initializer sets the initial values ofname
andage
when a new object is created.
Creating Objects in Swift
To use a class, you need to create an object (also called an instance). This is done by calling the class name followed by parentheses.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func displayDetails() {
print("Name: \(name), Age: \(age)")
}
}
let student = Student(name: "Kumar", age: 20)
student.displayDetails()
Explanation:
- Object creation: The
Student
class is instantiated using theStudent(name: "Kumar", age: 20)
initializer. - Method call: The
displayDetails
method is called using dot notation to print the student’s details.
Output:
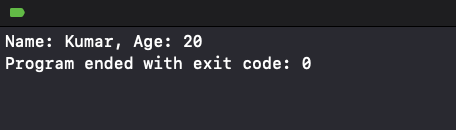
Accessing Properties and Methods
Properties and methods of a class object can be accessed using the dot operator (.
). This operator connects the object name to the property or method.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func displayDetails() {
print("Name: \(name), Age: \(age)")
}
}
let student = Student(name: "Arjun", age: 18)
// Accessing properties
print("Student Name: \(student.name)")
print("Student Age: \(student.age)")
// Accessing methods
student.displayDetails()
Explanation:
- Dot operator: Used to access
name
andage
properties as well as thedisplayDetails
method. - The
name
andage
properties are printed directly, while thedisplayDetails
method prints them through a formatted message.
Output:
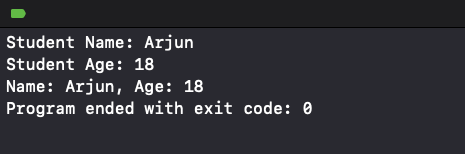
Classes as Reference Types
In Swift, classes are reference types. This means that when you assign a class object to another variable, both variables refer to the same instance. Any changes made through one variable will affect the other.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let student1 = Student(name: "Arjun", age: 22)
let student2 = student1
student2.name = "Ram"
print("Student1 Name: \(student1.name)") // Ram
print("Student2 Name: \(student2.name)") // Ram
Explanation:
- Reference type: Both
student1
andstudent2
refer to the same object in memory. - Changing the
name
property throughstudent2
also updates it forstudent1
.
Output:
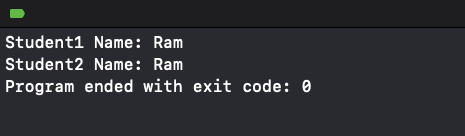
Conclusion
Classes in Swift provide a powerful way to model real-world entities and their behaviour.