Swift – Compare Strings
To compare Strings in Swift, we can use operators like greater than (>), less than (<), equal to (==), etc.
In this tutorial, we will go through the scenarios like, if a string is greater than the other, if a string is less than the other, or if two strings are equal.
String is Greater than Other String
To check if a string is greater than the other, use greater than operator as shown below.
str1 > str2
If string str1
is greater than string str2
lexicographically, then the greater than operator returns true
, else it returns false
.
Example
In the following program, we take two strings in variables str1
and str2
, and compare them using greater than operator.
main.swift
var str1 = "mango"
var str2 = "apple"
if (str1 > str2) {
print("\(str1) is greater than \(str2)")
} else {
print("\(str1) is not greater than \(str2)")
}
Output
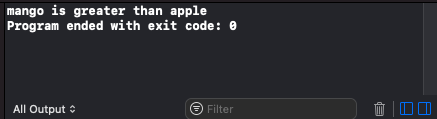
Now, let us take values for strings str1
and str2
, such that str1
is not greater than str2
.
main.swift
var str1 = "mango"
var str2 = "pears"
if (str1 > str2) {
print("\(str1) is greater than \(str2)")
} else {
print("\(str1) is not greater than \(str2)")
}
Output
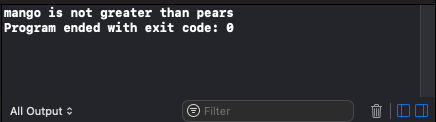
String is Less than Other String
To check if a string is less than the other, use less than operator as shown below.
str1 < str2
If string str1
is less than string str2
lexicographically, then the less than operator returns true
, else it returns false
.
Example
In the following program, we take two strings in variables str1
and str2
, and compare them using less than operator.
main.swift
var str1 = "apple"
var str2 = "mango"
if (str1 < str2) {
print("\(str1) is less than \(str2)")
} else {
print("\(str1) is not less than \(str2)")
}
Output
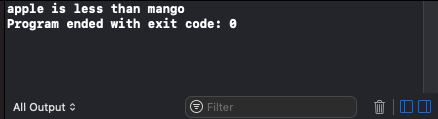
Now, let us take values for strings str1
and str2
, such that str1
is not less than str2
.
main.swift
var str1 = "pears"
var str2 = "mango"
if (str1 < str2) {
print("\(str1) is less than \(str2)")
} else {
print("\(str1) is not less than \(str2)")
}
Output
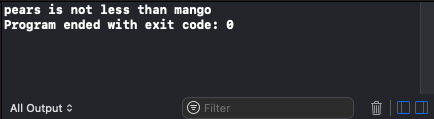
Strings are Equal
To check if a string is equal to the other, use equal to operator as shown below.
str1 == str2
If string str1
is equal to string str2
, then equal to operator returns true
, else it returns false
.
Example
In the following program, we take two strings in variables str1
and str2
, and compare them using equal to operator.
main.swift
var str1 = "mango"
var str2 = "mango"
if (str1 == str2) {
print("\(str1) is equal to \(str2)")
} else {
print("\(str1) is not equal to \(str2)")
}
Output
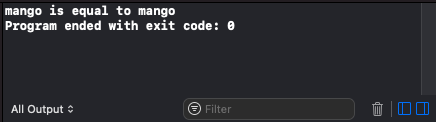
Now, let us take value for strings str1
and str2
, such that str1
is not equal to str2
.
main.swift
var str1 = "mango"
var str2 = "apple"
if (str1 == str2) {
print("\(str1) is equal to \(str2)")
} else {
print("\(str1) is not equal to \(str2)")
}
Output
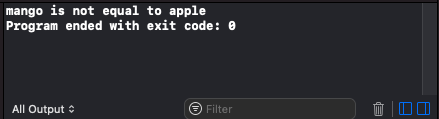
Conclusion
In this Swift Tutorial, we learned how to compare Strings in Swift, with examples.