Swift Comparison Operators
In Swift, comparison operators are used to compare two values. These operators return a Boolean value (true
or false
) based on whether the comparison is true or false. Comparison operators are commonly used in conditional statements and loops to make decisions in your program.
Comparison Operators in Swift
Here is a list of comparison operators available in Swift:
Operator | Description | Example |
---|---|---|
== | Equal to | 5 == 5 returns true |
!= | Not equal to | 5 != 3 returns true |
< | Less than | 3 < 5 returns true |
> | Greater than | 5 > 3 returns true |
<= | Less than or equal to | 3 <= 5 returns true |
>= | Greater than or equal to | 5 >= 5 returns true |
Example: Demonstrating All Comparison Operators
This example shows how each comparison operator works:
File: main.swift
let a = 10
let b = 20
print("a == b: \(a == b)") // false
print("a != b: \(a != b)") // true
print("a < b: \(a < b)") // true
print("a > b: \(a > b)") // false
print("a <= b: \(a <= b)") // true
print("a >= b: \(a >= b)") // false
Explanation:
a == b
: Checks ifa
is equal tob
. Returnsfalse
because10
is not equal to20
.a != b
: Checks ifa
is not equal tob
. Returnstrue
because10
is not equal to20
.a < b
: Checks ifa
is less thanb
. Returnstrue
because10
is less than20
.a > b
: Checks ifa
is greater thanb
. Returnsfalse
because10
is not greater than20
.a <= b
: Checks ifa
is less than or equal tob
. Returnstrue
because10
is less than20
.a >= b
: Checks ifa
is greater than or equal tob
. Returnsfalse
because10
is not greater than20
.
Output:
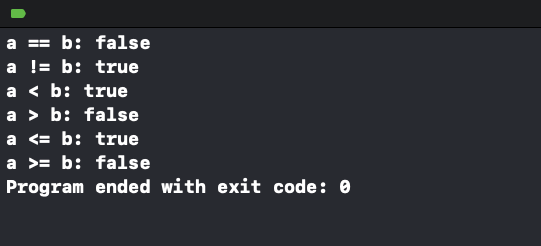
Use Cases of Comparison Operators
Example 1: Using Comparison Operators in Conditional Statements
Comparison operators are often used in if
statements to make decisions:
File: main.swift
let temperature = 30
if temperature > 25 {
print("It's a hot day!")
} else {
print("The weather is pleasant.")
}
Explanation:
- The
temperature
is compared to25
using the>
operator. - If the condition is true, the program prints “It’s a hot day!”; otherwise, it prints “The weather is pleasant.”
Output:
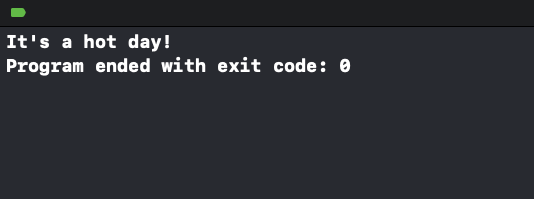
Example 2: Using Comparison Operators in Loops
Comparison operators are frequently used in loops to control iterations:
File: main.swift
for i in 1...5 {
if i % 2 == 0 {
print("\(i) is even")
} else {
print("\(i) is odd")
}
}
Explanation:
- The loop iterates through the numbers from
1
to5
. - The
%
operator checks if the number is divisible by2
. - The
==
operator is used to determine whether the remainder is equal to0
.
Output:
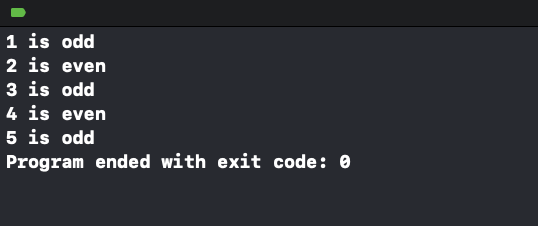
Conclusion
Comparison operators are essential for decision-making and controlling program flow in Swift. Understanding how to use these operators effectively will help you write more robust and dynamic code.