Constants in Swift Language
In Swift, constants are used to store data that should not change during the execution of a program. Unlike variables, constants have fixed values that cannot be modified after being set. These are essential for ensuring code safety and predictability.
Constants are declared using the let
keyword. They are ideal for values that should remain fixed throughout the program, such as mathematical constants or configuration settings.
Example 1: Declaring and Using Constants
This program demonstrates how to declare constants and use them in calculations:
File: main.swift
let gravity = 9.8
let mass = 50.0
// Calculate weight using the formula: weight = mass * gravity
let weight = mass * gravity
print("Mass: \(mass) kg")
print("Gravity: \(gravity) m/s²")
print("Weight: \(weight) N")
Explanation:
gravity
is declared as a constant with the value9.8
(the acceleration due to gravity).mass
is another constant with a value of50.0
kilograms.- The
weight
is calculated by multiplyingmass
andgravity
. - The
print
statements display the values ofmass
,gravity
, andweight
.
Output:
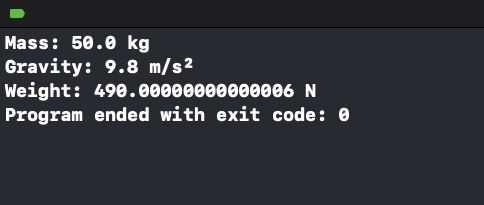
Example 2: Using Constants for Immutable Values
This example highlights the immutability of constants:
File: main.swift
let pi = 3.14159
print("Value of pi: \(pi)")
//updating the constant raises error
pi = 3.14
Explanation:
pi
is declared as a constant with the value3.14159
.- Since
pi
is a constant, attempting to modify it (as shown in the commented-out line) will result in a compile-time error.
Output:
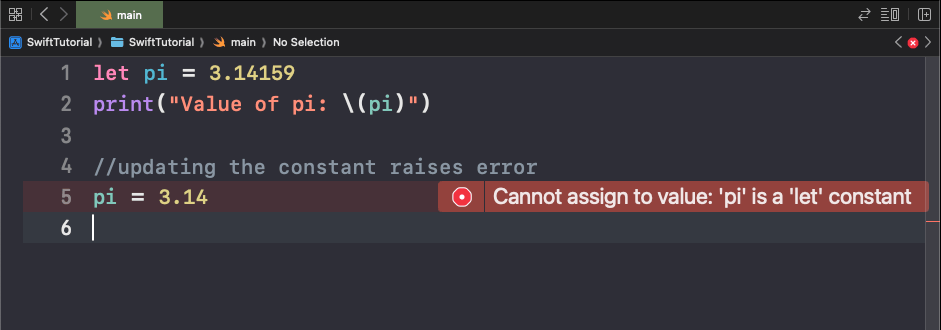
Example 3: Constants and Variables Together
This program demonstrates how constants and variables can be used together:
File: main.swift
let base = 10
var height = 5
// Calculate the area of a rectangle using the formula: area = base * height
let area = base * height
print("Base: \(base)")
print("Height: \(height)")
print("Area: \(area)")
// Update the height and recalculate the area
height = 7
let newArea = base * height
print("New Height: \(height)")
print("New Area: \(newArea)")
Explanation:
base
is declared as a constant because its value does not change.height
is a variable because its value is updated later in the program.- The
area
is calculated using the initial values ofbase
andheight
. - The height is updated to a new value, and the area is recalculated to reflect the change.
Output:
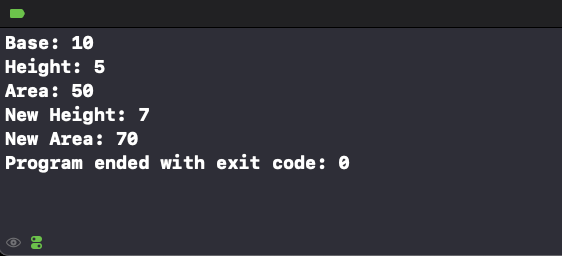
Benefits of Using Constants
Using constants improves the clarity and safety of your code:
- Immutability: Constants prevent accidental modifications to critical values.
- Readability: Constants convey intent by making it clear which values should remain unchanged.
- Optimization: The Swift compiler can optimize code with constants more effectively.
Conclusion
Constants are a key feature in Swift programming, enabling you to create safer and more predictable code. Use let
for values that should not change.