Data Types in Swift
Data types in Swift define the kind of values a variable or constant can hold. Swift is a type-safe language, meaning it ensures that the types of variables and constants are explicitly defined and used correctly.
Built-in Data Types
Swift provides several built-in data types for handling different kinds of data. Here are some common types:
Type | Description | Example |
---|---|---|
Int | Represents integer values | let age: Int = 25 |
Double | Represents decimal numbers with double-precision | let pi: Double = 3.14159 |
Float | Represents decimal numbers with single-precision | let temperature: Float = 36.6 |
String | Represents text | let greeting: String = "Hello, Swift!" |
Bool | Represents a Boolean value (true or false ) | let isSwiftFun: Bool = true |
In the following example, we define some variable with different data types, initialise them with values of the respective data types, and then print them to output.
File: main.swift
let age: Int = 30
let pi: Double = 3.14159
let temperature: Float = 25.5
let greeting: String = "Hello, Swift!"
let isSwiftFun: Bool = true
print("Age: \(age)")
print("Value of Pi: \(pi)")
print("Temperature: \(temperature)°C")
print("Greeting: \(greeting)")
print("Is Swift fun? \(isSwiftFun)")
Explanation:
Int
: Represents an integer value (e.g., age).Double
: Used for double-precision floating-point numbers (e.g., pi).Float
: Used for single-precision floating-point numbers (e.g., temperature).String
: Stores textual data (e.g., greeting).Bool
: Represents a Boolean value (true
orfalse
).
Output:
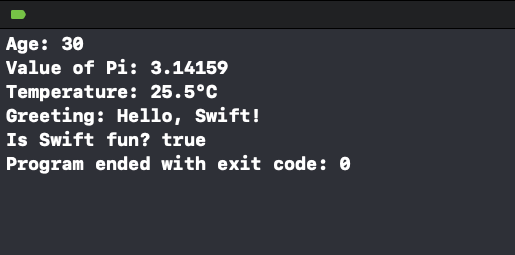
Bound Values
Bound values refer to the range of values a specific data type can hold:
Type | Minimum Value | Maximum Value |
---|---|---|
Int | -9223372036854775808 | 9223372036854775807 |
UInt | 0 | 18446744073709551615 |
Float | -3.4e38 | 3.4e38 |
Double | -1.7e308 | 1.7e308 |
File: main.swift
print("Int max: \(Int.max), Int min: \(Int.min)")
print("UInt max: \(UInt.max), UInt min: \(UInt.min)")
Output:
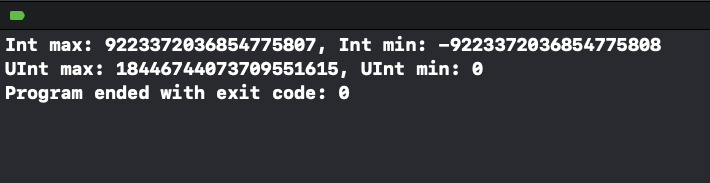
User-Defined Data Types
Swift allows you to define your own data types using structures, classes, and enumerations:
Type | Description | Example |
---|---|---|
Struct | Defines a value type that can encapsulate properties and methods. | struct Point { var x: Double; var y: Double } |
Class | Defines a reference type that can encapsulate properties, methods, and initializers. | class Vehicle { var make: String; var model: String } |
Enum | Defines a type with a finite set of possible values. | enum Direction { case north, south, east, west } |
File: main.swift
struct Point {
var x: Double
var y: Double
}
let point = Point(x: 3.0, y: 4.0)
print("Point: (\(point.x), \(point.y))")
Explanation:
Point
is a custom data type that represents a 2D point.- You can create instances of
Point
and access its properties.
Output:
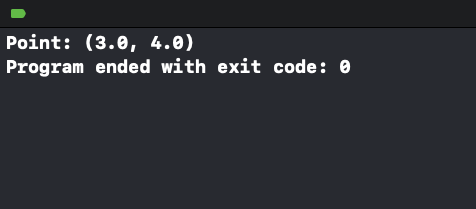
Defining Data Types
Defining your own data types provides flexibility and better code organization. For example:
File: main.swift
class Vehicle {
var make: String
var model: String
init(make: String, model: String) {
self.make = make
self.model = model
}
}
let car = Vehicle(make: "Tesla", model: "Model 3")
print("Car: \(car.make) \(car.model)")
Explanation:
- The
Vehicle
class represents a vehicle with a make and model. - You can create instances and initialize them with specific values.
Output:
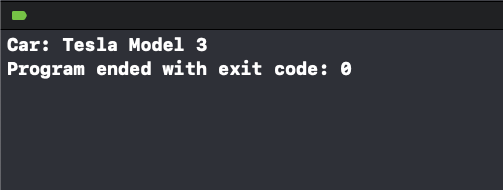
Type Safety in Swift
Swift enforces type safety, ensuring that variables and constants can only hold values of the declared type. This prevents runtime errors caused by type mismatches:
File: main.swift
let age: Int = 25
// age = "twenty-five" // Error: Cannot assign a String to an Int
Explanation:
- Type safety ensures that incorrect assignments are caught at compile time.
- This feature helps you write more reliable and predictable code.
Output:
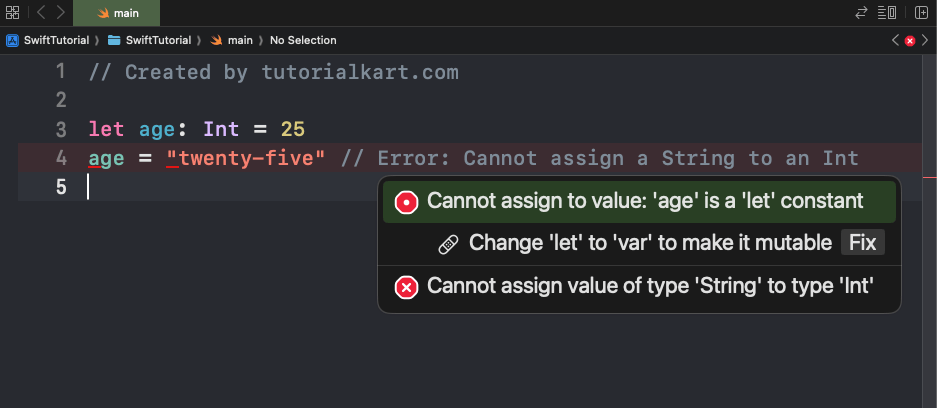
Type Inference in Swift
Swift can automatically infer the type of a variable or constant based on its initial value, reducing the need for explicit type annotations:
File: main.swift
let message = "Hello, Swift!" // Inferred as String
let number = 42 // Inferred as Int
let pi = 3.14 // Inferred as Double
Explanation:
- The type of each variable or constant is inferred based on its initial value.
- Type inference makes code cleaner and reduces redundancy.
Conclusion
Swift’s data types provide a robust foundation for writing safe and efficient code.