Swift Deinitialization
Deinitialization is the process of cleaning up and deallocating resources before an object is destroyed.
In Swift, deinitializers are used to perform this cleanup work. Deinitializers are called automatically just before an instance of a class is deallocated, making them useful for tasks like closing file handles, releasing memory, or other cleanup operations.
Deinitialization is only available for classes, as structures and enumerations are value types and do not require deinitializers. Each class can have at most one deinitializer, defined using the deinit
keyword.
Key Points:
- Deinitializers are called automatically when an object is no longer needed.
- They cannot take parameters and are declared without parentheses.
- Deinitializers are executed just before the memory occupied by an object is reclaimed.
Syntax
The syntax for defining a deinitializer is straightforward:
class ClassName {
deinit {
// Cleanup code
}
}
Example of a Deinitializer
Let’s create a class that uses a deinitializer to perform cleanup operations:
Example: main.swift
class FileHandler {
var fileName: String
init(fileName: String) {
self.fileName = fileName
print("Opening file: \(fileName)")
}
deinit {
print("Closing file: \(fileName)")
}
}
func manageFile() {
let handler = FileHandler(fileName: "example.txt")
// File operations
}
manageFile()
print("End of program.")
Explanation:
- The
FileHandler
class initializes with afileName
and prints a message when the file is opened. - The
deinit
method prints a message when the object is deallocated, simulating the closing of a file. - The
manageFile
function creates aFileHandler
instance, and the deinitializer is called automatically when the function scope ends.
Output:
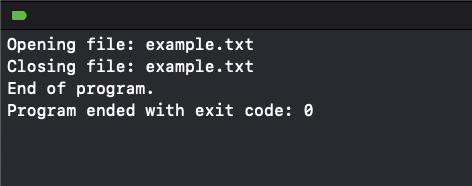
Use Cases for Deinitializers
Deinitializers are commonly used for:
- Releasing system resources like file handles, sockets, or database connections.
- Cleaning up manually allocated memory or resources not managed by Swift’s ARC (Automatic Reference Counting).
- Logging the lifecycle of objects for debugging purposes.
Important Notes
- Deinitializers are not explicitly called in code; they are managed by the system.
- They are only available in classes and cannot be inherited or overridden.
- Ensure that cleanup tasks in the
deinit
block are efficient, as it is the final step before memory deallocation.
Conclusion
Deinitialization is a crucial part of managing resources in object-oriented programming with Swift. By using deinitializers, you can ensure that resources are cleaned up properly when an object is no longer needed.