In this tutorial, we will go through Swift programs to find the factorial of a given number, in different approaches like using for-in loop, recursion, while loop, etc.
Swift Factorial Program using For-in Loop
In the following program, we will use for-in loop for computing factorial of a given number.
main.swift
</>
Copy
func factorial(n: Int) -> Int {
var result = 1
if(n > 0) {
for i in 1...n {
result *= i
}
}
return result
}
let num = 4
let result = factorial(n: num)
print("\(num)! = \(result)")
Output
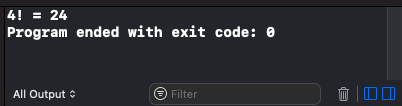
Swift Factorial Program using Recursion
In the following program, we will use recursion mechanism for computing factorial of a given number.
main.swift
</>
Copy
func factorial(n: Int) -> Int {
return n <= 1 ? 1 : n * factorial(n: n - 1)
}
let num = 5
let result = factorial(n: num)
print("\(num)! = \(result)")
Output
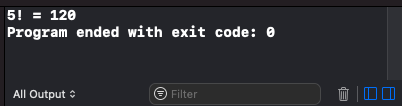
Swift Factorial Program using While Loop
In the following program, we will use while loop for computing factorial of a given number.
main.swift
</>
Copy
func factorial(n: Int) -> Int {
var result = 1
var i = 1
while i <= n {
result *= i
i += 1
}
return result
}
let num = 5
let result = factorial(n: num)
print("\(num)! = \(result)")
Output
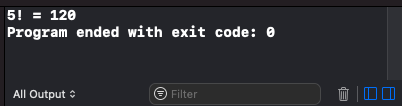
Conclusion
In this Swift Tutorial, we learned how to find the factorial of a given number using for-in statement, recursion, etc., with examples.