Swift Floating Point Numbers
Floating-point numbers are used to represent numbers with fractional components. Swift provides two main types of floating-point numbers: Float
and Double
. These types differ in precision and range, allowing developers to choose the most appropriate type for their needs.
Types of Floating-Point Numbers in Swift
Here’s a comparison of Swift’s floating-point types:
Type | Size (bits) | Precision | Range |
---|---|---|---|
Float | 32 | 6-7 decimal digits | ~ -3.4e38 to 3.4e38 |
Double | 64 | 15-16 decimal digits | ~ -1.7e308 to 1.7e308 |
Using Floating-Point Numbers in Swift
Here’s an example demonstrating the use of floating-point numbers:
File: main.swift
let pi: Double = 3.14159265359
let temperature: Float = 36.6
print("Value of Pi: \(pi)")
print("Temperature: \(temperature)°C")
Explanation:
Double
: Used for storing high-precision decimal numbers, such aspi
.Float
: Used for storing lower-precision decimal numbers, such astemperature
.
Output:
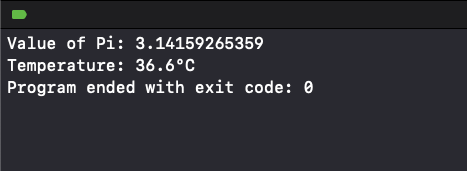
Precision Differences
Here’s an example illustrating the difference in precision between Float
and Double
:
File: main.swift
let floatValue: Float = 0.123456789
let doubleValue: Double = 0.1234567890123456789
print("Float value: \(floatValue)")
print("Double value: \(doubleValue)")
Explanation:
Float
: Loses precision after 6-7 decimal places.Double
: Maintains precision up to 15-16 decimal places.
Output:
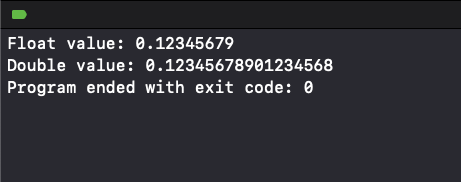
Using Floating Point Numbers in Arithmetic Operations
Floating-point numbers can be used in arithmetic operations. Here’s an example:
File: main.swift
let radius: Double = 5.0
let area = Double.pi * radius * radius
print("Area of the circle: \(area)")
Explanation:
- The constant
Double.pi
provides the value ofπ
. - The formula for the area of a circle,
πr²
, is applied usingradius
.
Output:
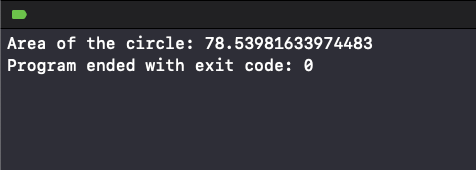
When to Use Float vs. Double
Here are some guidelines for choosing between Float
and Double
:
- Use
Double
: When high precision is required (e.g., scientific calculations). - Use
Float
: When memory efficiency is more important than precision (e.g., graphics programming).
Conclusion
Floating-point numbers are essential for representing decimal and fractional values in Swift. By understanding the differences between Float
and Double
, you can make informed decisions on which type to use for your specific needs.