Swift Function – Default Value for Parameter(s)
To assign default values for parameter(s) of a function in Swift, assign the parameter(s) with the required default value in the function definition.
The syntax to specify a default value for parameter of function is
func functionName(parameterName: Type = defaultValue) { //body }
Example
In the following program, we will define a function add()
with two parameters whose default value is 0.
main.swift
func add(a: Int = 0, b: Int = 0) { print(a+b) } add(a: 10, b: 20) add(a: 5) add(b: 4)
Output
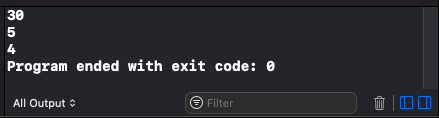
For add(a: 10, b: 20)
, we have passed values for both the parameters.
For add(a: 5)
, we have passed value only for the parameter a
. In this case, b
would be considered 0
since the default value of b
is 0
.
Similarly, for add(b: 4)
, we have passed value only for the parameter b
. In this case, a
would be considered 0
.
Now, let us define a function greet()
that prints a greeting message to user. If accepts a String parameter. If no value is passed, it should consider the user name as just User
. For this scenario, we will assign the default of name
parameter to be User
.
main.swift
func greet(name: String = "User") { print("Hello \(name)!") } greet() greet(name: "Abc")
Output
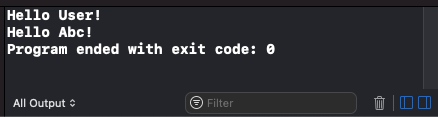
Conclusion
In this Swift Tutorial, we learned how to assign a default value for parameter(s) in function definition.