Identity Operators in Swift
In Swift, identity operators are used to check whether two objects reference the same instance in memory. These operators are useful for comparing instances of classes and ensuring that they are identical in reference, not just in value. Swift provides two identity operators:
===
: Returnstrue
if two references point to the same instance.!==
: Returnstrue
if two references do not point to the same instance.
Example: Using Identity Operators
In this example, we will demonstrate how the identity operators work with class instances. The program creates two separate instances of a class and compares them using identity operators to check if they refer to the same object.
File: main.swift
class Person {
var name: String
init(name: String) {
self.name = name
}
}
let person1 = Person(name: "Alice")
let person2 = Person(name: "Alice")
let person3 = person1
// Identity comparison
print("person1 === person2: \(person1 === person2)") // false
print("person1 === person3: \(person1 === person3)") // true
print("person1 !== person2: \(person1 !== person2)") // true
Explanation:
person1
andperson2
are two separate instances of thePerson
class, even though they have the same property values.- The identity operator
===
checks if two references point to the same instance. Sinceperson1
andperson2
are different instances, the comparison returnsfalse
. person3
is assigned toperson1
, so they refer to the same instance. The comparisonperson1 === person3
returnstrue
.- The non-identity operator
!==
confirms thatperson1
andperson2
are not the same instance, returningtrue
.
Output:
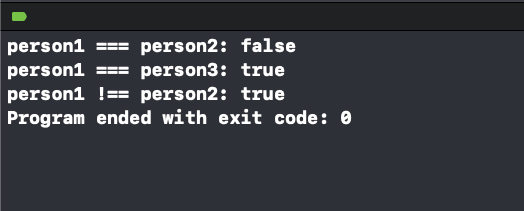
Example 1: Checking Identity in Conditional Statements
In this example, the program uses identity operators in a conditional statement to determine whether two objects are the same.
File: main.swift
class Car {
var model: String
init(model: String) {
self.model = model
}
}
let car1 = Car(model: "Tesla Model S")
let car2 = Car(model: "Tesla Model S")
let car3 = car1
if car1 === car3 {
print("car1 and car3 refer to the same instance.")
} else {
print("car1 and car3 do not refer to the same instance.")
}
if car1 !== car2 {
print("car1 and car2 refer to different instances.")
}
Explanation:
- The program creates three
Car
objects:car1
,car2
, andcar3
. car1
andcar3
refer to the same instance, so the conditional statement prints that they refer to the same instance.car1
andcar2
are different instances, so the second conditional statement prints that they refer to different instances.
Output:
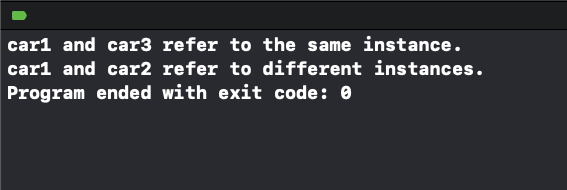
Conclusion
Swift’s identity operators are crucial for comparing references of class instances. They allow you to determine whether two variables point to the same object in memory, making them essential for managing object identity in your code.