Swift Inequality
To check if two values are not equal in Swift, we can use inequality operator.
Inequality Operator !=
takes two values as operands, one on the left and one on the right, and returns boolean value.
The return value is true if the two operands are not equal, else it returns false.
The syntax to check if two values a
and b
are not equal is
a != b
Examples
In the following program, we will use Inequality Operator and check if two strings are not equal.
main.swift
var a = "Hello World"
var b = "Good Morning"
var result = a != b
print("Are the two values not equal? \(result)")
Output
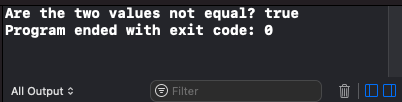
Now, let us check if two numbers are not equal using Inequality Operator.
main.swift
var a = 25
var b = 16
var result = a != b
print("Are the two values not equal? \(result)")
Output
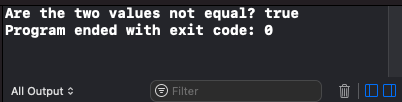
Since the Inequality Operator returns boolean value, we can use this expression as condition in if statement.
main.swift
var a = 25
var b = 16
if a != b {
print("The two values are not equal.")
} else {
print("The two values are equal.")
}
Output
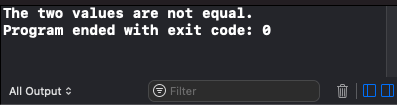
Conclusion
In this Swift Tutorial, we learned how to perform Inequality operation in Swift programming.