Swift Inheritance
Inheritance is a fundamental concept in object-oriented programming, allowing one class (the subclass) to inherit properties, methods, and behaviour from another class (the base class).
Basics of Inheritance
Base Class
A base class is the parent class from which other classes can inherit. It provides common properties and methods that can be used or overridden by its subclasses.
Base class is also called parent class or super class, and are used interchangeably.
Example: main.swift
class Animal {
var name: String
init(name: String) {
self.name = name
}
func makeSound() {
print("\(name) makes a sound.")
}
}
Subclass
A subclass is a child class that inherits from a base class. It can add its own properties and methods or override those of the base class.
Example: main.swift
class Animal {
var name: String
init(name: String) {
self.name = name
}
func makeSound() {
print("\(name) makes a sound.")
}
}
class Dog: Animal {
func bark() {
print("\(name) barks.")
}
}
let dog = Dog(name: "Buddy")
dog.makeSound() // Inherited from Animal
dog.bark() // Defined in Dog
Output:
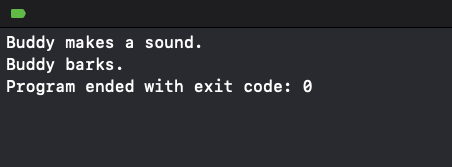
Is-A Relationship
The inheritance model in Swift is based on the “is-a” relationship. This means that a subclass is a specialized version of its superclass. For example:
- A dog “is an” animal, so it inherits from the
Animal
class. - A cat “is an” animal, so it could also inherit from the
Animal
class.
Method Overriding
Method overriding allows a subclass to provide its own implementation of a method that is defined in its superclass. The override
keyword is used to indicate that a method is being overridden.
Example: main.swift
class Animal {
func makeSound() {
print("An animal makes a sound.")
}
}
class Cat: Animal {
override func makeSound() {
print("The cat meows.")
}
}
let animal = Animal()
animal.makeSound()
let cat = Cat()
cat.makeSound()
Output:
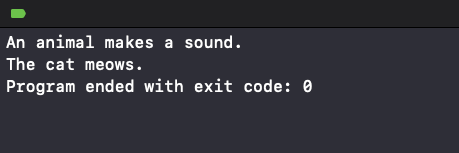
Using the super
Keyword
The super
keyword is used in a subclass to call methods or access properties of its superclass. This is particularly useful when overriding methods and you want to include the superclass’s implementation.
Example: main.swift
class Animal {
func makeSound() {
print("An animal makes a sound.")
}
}
class Dog: Animal {
override func makeSound() {
super.makeSound()
print("The dog barks.")
}
}
let dog = Dog()
dog.makeSound()
Output:
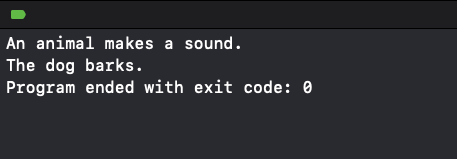
Types of Inheritance
1. Single Inheritance
In single inheritance, a subclass inherits from a single base class.
Example: main.swift
class Vehicle {
func move() {
print("The vehicle moves.")
}
}
class Car: Vehicle {
func drive() {
print("The car drives.")
}
}
let car = Car()
car.move()
car.drive()
Output:
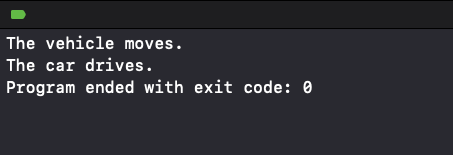
2. Multi-Level Inheritance
In multi-level inheritance, a class inherits from a class that is already a subclass.
Example: main.swift
class Vehicle {
func move() {
print("The vehicle moves.")
}
}
class Car: Vehicle {
func drive() {
print("The car drives.")
}
}
class SportsCar: Car {
func race() {
print("The sports car races.")
}
}
let sportsCar = SportsCar()
sportsCar.move()
sportsCar.drive()
sportsCar.race()
Output:
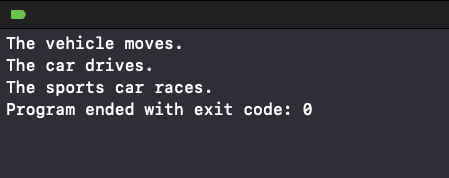
3. Hybrid Inheritance
Hybrid inheritance combines single and multi-level inheritance to create a complex hierarchy. While Swift does not support multiple inheritance (a class cannot inherit from multiple classes), hybrid patterns can be achieved using protocols.
Example: Achieving hybrid behavior with protocols.
Example: main.swift
protocol Flyable {
func fly()
}
class Bird {
func layEggs() {
print("The bird lays eggs.")
}
}
class Parrot: Bird, Flyable {
func fly() {
print("The parrot flies.")
}
}
let parrot = Parrot()
parrot.layEggs()
parrot.fly()
Output:
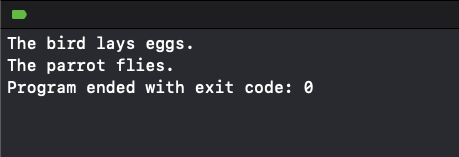
Need for Inheritance
Inheritance provides several benefits in programming:
- Code Reusability: Common properties and methods are defined once in a base class and reused by subclasses.
- Extensibility: Subclasses can extend or modify the behavior of the base class.
- Polymorphism: Subclasses can override methods to provide specific behaviour while maintaining a consistent interface.
- Organization: Inheritance helps organize related classes into a hierarchical structure.