Swift Initializers
Initializers are special methods in Swift that prepare an instance of a class, structure, or enumeration for use. They are responsible for initializing properties and performing any necessary setup when a new instance is created. Swift provides a variety of initializers, including custom initializers, convenience initializers, and more.
What is an Initializer?
An initializer is a function-like method that has no return value. It is declared using the init
keyword and is automatically called when a new instance of a type is created. Initializers ensure that all properties of an instance are properly initialized before it is used.
Syntax:
init(parameters) {
// Initialization code
}
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let student = Student(name: "Arjun", age: 20)
print("Name: \(student.name), Age: \(student.age)")
Explanation:
- The
init
method initializes thename
andage
properties when a newStudent
instance is created. - The
self
keyword is used to distinguish between property names and parameter names.
Output:
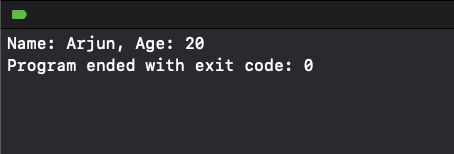
Default Initializers
If all properties in a type have default values, Swift automatically provides a default initializer that requires no arguments.
Example: main.swift
class Car {
var brand: String = "Unknown"
var year: Int = 2023
}
let car = Car()
print("Brand: \(car.brand), Year: \(car.year)")
Explanation:
- The
Car
class has a default initializer because all properties have default values.
Output:
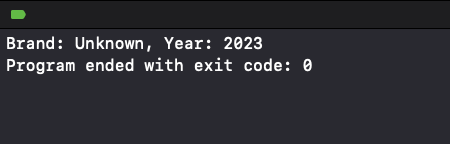
Custom Initializers
Custom initializers allow you to provide specific initialization logic or accept parameters to initialize properties.
Example: main.swift
class Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
}
let rect = Rectangle(width: 10.0, height: 20.0)
print("Width: \(rect.width), Height: \(rect.height)")
Explanation:
- The
Rectangle
class has a custom initializer to accept width and height values as parameters.
Output:
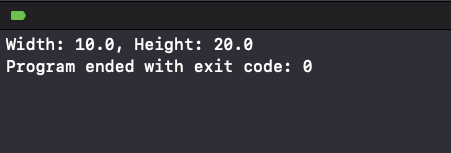
Convenience Initializers
Convenience initializers are secondary initializers that delegate the initialization to a designated initializer. They are defined using the convenience
keyword.
Example: main.swift
class Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
convenience init(side: Double) {
self.init(width: side, height: side)
}
}
let square = Rectangle(side: 10.0)
print("Width: \(square.width), Height: \(square.height)")
Explanation:
- The
side
parameter is used to create a square by initializing both width and height to the same value. - The
convenience
initializer delegates initialization to the designated initializer.
Output:
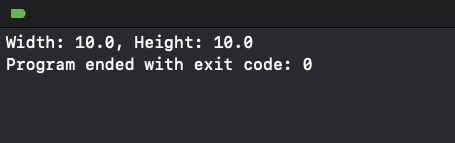
Failable Initializers
Failable initializers can return nil
if initialization fails. They are declared using the init?
keyword.
Example: main.swift
class Person {
var name: String
var age: Int
init?(name: String, age: Int) {
if age < 0 {
return nil
}
self.name = name
self.age = age
}
}
if let person = Person(name: "Bob", age: -5) {
print("Name: \(person.name), Age: \(person.age)")
} else {
print("Invalid age provided.")
}
Explanation:
- The initializer returns
nil
if the age is less than 0, indicating that the object could not be created.
Output:
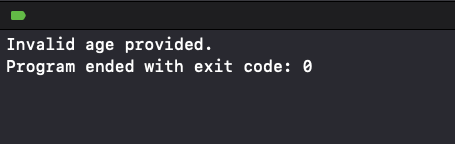
Required Initializers
A required
initializer ensures that every subclass implements the initializer. It is declared using the required
keyword.
Example: main.swift
class Animal {
var name: String
required init(name: String) {
self.name = name
}
}
class Dog: Animal {
required init(name: String) {
super.init(name: name)
}
}
let dog = Dog(name: "Buddy")
print("Dog's name: \(dog.name)")
Explanation:
- The
required
keyword ensures that subclasses provide their own implementation of the initializer.
Output:
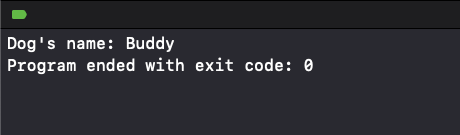
Conclusion
Swift initializers are a powerful feature that ensures objects are created with valid data. By mastering different types of initializers—default, custom, convenience, failable, and required—you can write robust and flexible Swift programs.