Swift Integers
Integers are a fundamental data type in Swift, representing whole numbers without any fractional component. Swift provides a variety of integer types to suit different use cases, allowing for both signed and unsigned values with varying sizes.
Swift Integer Types
Swift includes several integer types, each with specific size and range. Here’s an overview:
Type | Size (bits) | Range |
---|---|---|
Int | Platform-dependent (32-bit or 64-bit) | -2^(n-1) to 2^(n-1) - 1 |
UInt | Platform-dependent (32-bit or 64-bit) | 0 to 2^n - 1 |
Int8 | 8 | -128 to 127 |
UInt8 | 8 | 0 to 255 |
Int16 | 16 | -32,768 to 32,767 |
UInt16 | 16 | 0 to 65,535 |
Int32 | 32 | -2,147,483,648 to 2,147,483,647 |
UInt32 | 32 | 0 to 4,294,967,295 |
Int64 | 64 | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
UInt64 | 64 | 0 to 18,446,744,073,709,551,615 |
Using Integers in Swift
Here’s an example of how different integer types are used in Swift:
File: main.swift
</>
Copy
let age: Int = 30
let maxUInt8: UInt8 = 255
let temperature: Int16 = -40
let population: UInt64 = 7_874_965_825
print("Age: \(age)")
print("Maximum value for UInt8: \(maxUInt8)")
print("Temperature: \(temperature)°C")
print("World population: \(population)")
Explanation:
Int
: Stores signed integers, adapting to the platform size.UInt8
: Stores unsigned 8-bit integers, ideal for small positive values.Int16
: Stores signed 16-bit integers, useful for small positive or negative values.UInt64
: Stores large unsigned integers, perfect for representing large positive values.
Output:
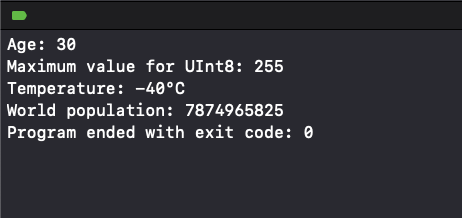
Overflow Behavior
Swift provides arithmetic overflow operators to handle cases where operations exceed the limits of an integer type. For example:
File: main.swift
</>
Copy
let maxValue: UInt8 = 255
let overflowedValue = maxValue &+ 1
print("Value after overflow: \(overflowedValue)")
Explanation:
- The value of
maxValue
is the maximum for aUInt8
type. - The
&+
operator performs addition but wraps around on overflow.
Output:
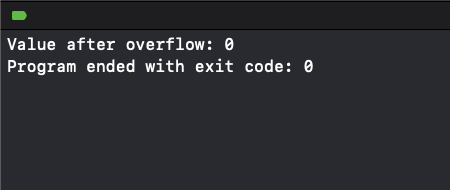
Conclusion
Understanding Swift’s integer types allows you to choose the right type for your specific needs, balancing memory efficiency and range requirements.