Swift Logical Operators
In Swift, logical operators are used to combine or invert Boolean values. They play a critical role in decision-making and controlling the flow of programs. Logical operators work with true
and false
values to form more complex conditions.
Table – Logical Operators in Swift
Here is a list of logical operators available in Swift:
Operator | Description | Example |
---|---|---|
&& | Logical AND | Returns true if both operands are true |
|| | Logical OR | Returns true if at least one operand is true |
! | Logical NOT | Inverts the Boolean value |
Example: Using Logical Operators
This example demonstrates how each logical operator works:
File: main.swift
let isSunny = true
let isWarm = false
// Logical AND
print("Is it sunny and warm? \(isSunny && isWarm)") // false
// Logical OR
print("Is it sunny or warm? \(isSunny || isWarm)") // true
// Logical NOT
print("Is it not sunny? \(!isSunny)") // false
Explanation:
- The
&&
operator checks if bothisSunny
andisWarm
aretrue
. SinceisWarm
isfalse
, the result isfalse
. - The
||
operator checks if eitherisSunny
orisWarm
istrue
. SinceisSunny
istrue
, the result istrue
. - The
!
operator inverts the value ofisSunny
. SinceisSunny
istrue
, the result isfalse
.
Output:
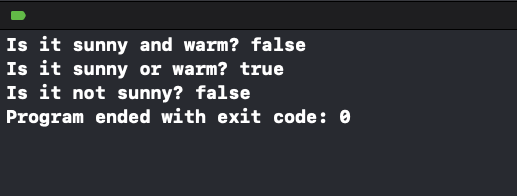
Example 1: Combining Conditions
This example shows how logical operators can combine multiple conditions:
File: main.swift
let age = 20
let hasID = true
if age >= 18 && hasID {
print("You are allowed to enter.")
} else {
print("You are not allowed to enter.")
}
Explanation:
- The condition
age >= 18 && hasID
checks if the person is at least 18 years old and has an ID. - Since both conditions are
true
, the program prints “You are allowed to enter.”
Output:
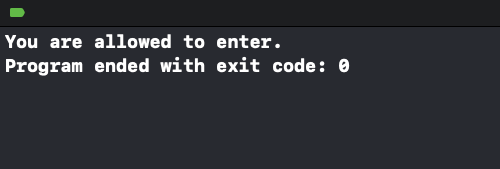
Example 2: Using Logical OR
This example demonstrates the use of the ||
operator:
File: main.swift
let hasKey = false
let knowsPassword = true
if hasKey || knowsPassword {
print("You can unlock the door.")
} else {
print("Access denied.")
}
Explanation:
- The condition
hasKey || knowsPassword
checks if the person either has the key or knows the password. - Since
knowsPassword
istrue
, the program prints “You can unlock the door.”
Output:
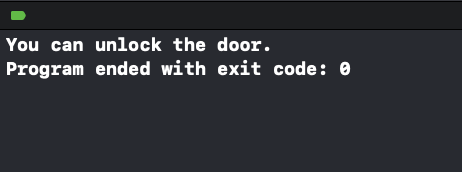
Example 3: Using Logical NOT
This example demonstrates the !
operator:
File: main.swift
let isOnline = false
if !isOnline {
print("The user is offline.")
} else {
print("The user is online.")
}
Explanation:
- The
!
operator inverts the value ofisOnline
. - Since
isOnline
isfalse
,!isOnline
evaluates totrue
, and the program prints “The user is offline.”
Output:
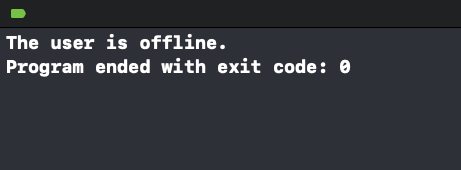
Conclusion
Logical operators are essential tools for combining and manipulating Boolean values in Swift. They are widely used in conditional statements and loops to implement complex logic.