Swift Method Overriding
Method overriding is a fundamental feature of object-oriented programming that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. In Swift, method overriding enables developers to modify or extend the behaviour of inherited methods, making classes more flexible and adaptable.
What is Method Overriding?
Method overriding occurs when a subclass provides its own implementation of a method that is defined in its superclass. The overriding method must have the same name, parameters, and return type as the method in the superclass. The override
keyword is used to indicate that a method is being overridden.
Syntax:
class Superclass {
func methodName() {
// Original implementation
}
}
class Subclass: Superclass {
override func methodName() {
// Overridden implementation
}
}
Declaring a Superclass and Overriding Methods
Let’s define a superclass Student
with a method displayDetails
, and then override this method in a subclass GraduateStudent
.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func displayDetails() {
print("Name: \(name), Age: \(age)")
}
}
class GraduateStudent: Student {
var degree: String
init(name: String, age: Int, degree: String) {
self.degree = degree
super.init(name: name, age: age)
}
override func displayDetails() {
print("Name: \(name), Age: \(age), Degree: \(degree)")
}
}
let gradStudent = GraduateStudent(name: "Arjun", age: 24, degree: "M.Sc")
gradStudent.displayDetails()
Explanation:
- The
Student
class defines thedisplayDetails
method to print the student’s name and age. - The
GraduateStudent
subclass overrides thedisplayDetails
method to include the degree information. - The
super
keyword is used to call the superclass initializer.
Output:
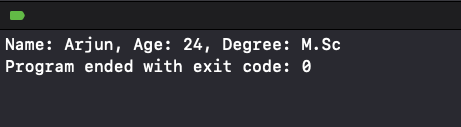
Accessing Superclass Methods
Even when a method is overridden, you can still access the superclass implementation using the super
keyword.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func displayDetails() {
print("Name: \(name), Age: \(age)")
}
}
class GraduateStudent: Student {
var degree: String
init(name: String, age: Int, degree: String) {
self.degree = degree
super.init(name: name, age: age)
}
override func displayDetails() {
super.displayDetails()
print("Degree: \(degree)")
}
}
let gradStudent = GraduateStudent(name: "Ram", age: 26, degree: "MBA")
gradStudent.displayDetails()
Explanation:
- The
super.displayDetails()
call invokes thedisplayDetails
method of theStudent
superclass. - Additional functionality is added in the overridden method to print the degree.
Output:
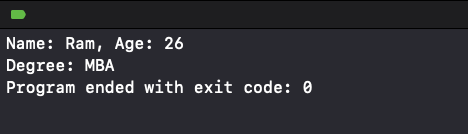
Rules for Method Overriding
- The method in the subclass must use the
override
keyword. - The overriding method must have the same name, parameters, and return type as the method in the superclass.
- The superclass method being overridden must be accessible (e.g., not marked as
private
).
Why Use Method Overriding?
Method overriding is useful for:
- Customizing behavior in subclasses.
- Adding additional functionality while preserving the base implementation.
- Creating polymorphic behavior where objects of different subclasses can be treated uniformly but exhibit different behavior.