Swift Methods
Methods in Swift are functions that are associated with a particular type, such as a class, structure, or enumeration. They define behaviours that an object can perform or provide functionality related to a type. Swift supports several kinds of methods, including instance methods, static methods, mutating methods, and type methods, each serving specific purposes.
Basics of Methods
Instance methods are functions defined inside a class, structure, or enumeration. They operate on the instance of the type they belong to and can access and modify its properties.
Syntax:
class ClassName {
func methodName(parameters) -> ReturnType {
// Method body
}
}
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func greet() {
print("Hello, my name is \(name).")
}
}
let student = Student(name: "Arjun", age: 20)
student.greet()
Explanation:
- The
greet
method prints a greeting using thename
property. - Methods are called using the dot operator (
.
).
Output:
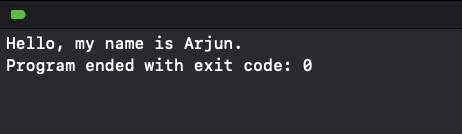
Static Methods
Static methods belong to the type itself rather than an instance. They are defined using the static
keyword.
Example: main.swift
class Math {
static func square(of number: Int) -> Int {
return number * number
}
}
let result = Math.square(of: 5)
print("Square: \(result)")
Explanation:
- The
square
method is a static method, accessed using the type name (Math.square
). - It calculates the square of a given number.
Output:
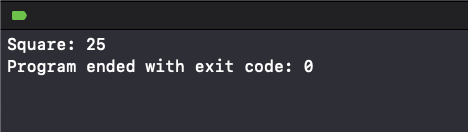
Self Property in Methods
The self
property refers to the instance of the type within its methods. It is used explicitly to disambiguate property names or pass the current instance as a parameter.
Example: main.swift
class Student {
var name: String
init(name: String) {
self.name = name
}
func introduce() {
print("This is \(self.name).")
}
}
let student = Student(name: "Ram")
student.introduce()
Explanation:
The self
property is used to explicitly refer to the name
property of the instance.
Output:
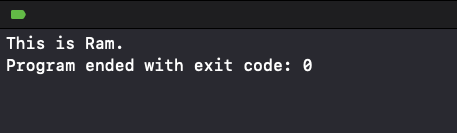
Mutating Methods
Structures and enumerations in Swift are value types. By default, methods of value types cannot modify their properties. To allow modification, you use the mutating
keyword.
Example: main.swift
struct Rectangle {
var width: Double
var height: Double
mutating func resize(toWidth width: Double, andHeight height: Double) {
self.width = width
self.height = height
}
}
var rect = Rectangle(width: 5.0, height: 10.0)
rect.resize(toWidth: 8.0, andHeight: 12.0)
print("Width: \(rect.width), Height: \(rect.height)")
Explanation:
The mutating
keyword allows the resize
method to modify the width
and height
properties.
Output:
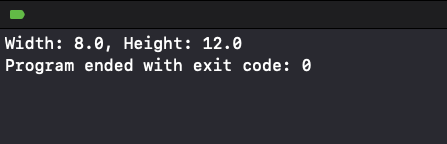
Type Methods
Type methods are defined using the static
or class
keyword. They are called on the type itself rather than on an instance. The class
keyword allows the method to be overridden by subclasses.
Example: main.swift
class Animal {
class func sound() {
print("Animals make sounds.")
}
}
class Dog: Animal {
override class func sound() {
print("Dogs bark.")
}
}
Animal.sound()
Dog.sound()
Explanation:
- The
sound
method inAnimal
is a type method defined with theclass
keyword. - The
Dog
subclass overrides thesound
method.
Output:
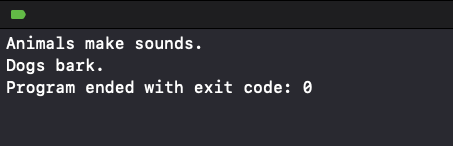
Differences Between Methods
Regular Method vs. Static Method
Feature | Regular Method | Static Method |
---|---|---|
Belongs to | Instance of the type | The type itself |
Access | Accessed using an instance | Accessed using the type name |
Usage | Used to operate on instance-specific data | Used for operations that are independent of any instance |
Static Method vs. Type Method
Feature | Static Method | Type Method |
---|---|---|
Keyword | static | class |
Inheritance | Cannot be overridden by subclasses | Can be overridden by subclasses |
Usage | Used for type-specific operations | Used for type-specific operations with support for inheritance |