Operator Overloading in Swift
In Swift, operator overloading allows you to define custom behaviour for existing operators when applied to your own types. This enables you to perform operations on your custom types using familiar syntax, such as +
, -
, or *
. With operator overloading, you can make your code more intuitive and expressive.
What Is Operator Overloading?
Operator overloading is the process of redefining the functionality of existing operators for user-defined types. Swift allows you to overload arithmetic, comparison, and other operators for structures, classes, and enumerations.
To overload an operator, you define a global function with the operator
keyword and implement the desired functionality for your custom type.
Example: Overloading the Addition Operator
This example demonstrates how to overload the +
operator for a custom structure:
File: main.swift
struct Vector {
var x: Double
var y: Double
// Overload the + operator
static func + (lhs: Vector, rhs: Vector) -> Vector {
return Vector(x: lhs.x + rhs.x, y: lhs.y + rhs.y)
}
}
let vector1 = Vector(x: 1.0, y: 2.0)
let vector2 = Vector(x: 3.0, y: 4.0)
let result = vector1 + vector2
print("Result: (\(result.x), \(result.y))")
Explanation:
- The
Vector
structure represents a 2D vector withx
andy
coordinates. - The
+
operator is overloaded using a static function. It takes twoVector
instances as input and returns a newVector
with coordinates added together. - The program creates two vectors and adds them using the overloaded
+
operator.
Output:
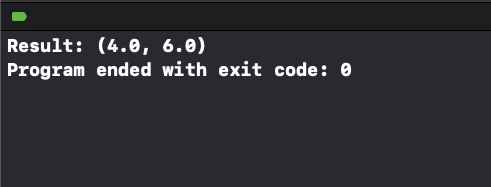
Example 1: Overloading the Multiplication Operator
This example demonstrates overloading the *
operator for scalar multiplication:
File: main.swift
struct Vector {
var x: Double
var y: Double
// Overload the * operator for scalar multiplication
static func * (lhs: Vector, rhs: Double) -> Vector {
return Vector(x: lhs.x * rhs, y: lhs.y * rhs)
}
}
let vector = Vector(x: 4.0, y: 6.0)
let scalar = 2.0
let scaledVector = vector * scalar
print("Scaled Vector: (\(scaledVector.x), \(scaledVector.y))")
Explanation:
- The
*
operator is overloaded to multiply a vector by a scalar (aDouble
value). - The function returns a new
Vector
with coordinates scaled by the scalar.
Output:
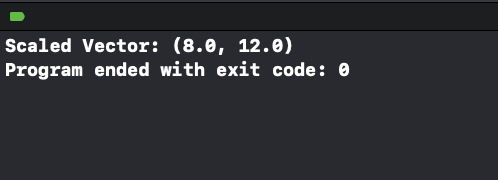
Example 2: Overloading the Equality Operator
This example demonstrates how to overload the ==
operator for equality comparison:
File: main.swift
struct Vector {
var x: Double
var y: Double
// Overload the == operator for equality comparison
static func == (lhs: Vector, rhs: Vector) -> Bool {
return lhs.x == rhs.x && lhs.y == rhs.y
}
}
let vector1 = Vector(x: 1.0, y: 2.0)
let vector2 = Vector(x: 1.0, y: 2.0)
let vector3 = Vector(x: 3.0, y: 4.0)
print("Vector1 == Vector2: \(vector1 == vector2)")
print("Vector1 == Vector3: \(vector1 == vector3)")
Explanation:
- The
==
operator is overloaded to compare twoVector
instances for equality. - The function checks whether the
x
andy
coordinates of both vectors are equal.
Output:
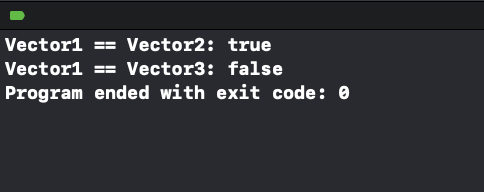
Conclusion
Operator overloading in Swift allows you to extend the functionality of operators to work seamlessly with your custom types. By overloading operators, you can write more intuitive and expressive code that aligns with your application’s requirements.