Swift Operators
In this tutorial, we will learn about different operators in Swift programming language.
Operators in Swift language can be grouped into following categories based on the type of operation they perform.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Identity Operators
- Bitwise Operators
In this tutorial, we will go through each of these categories, but, the following tutorials cover these in detail.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Identity Operators
- Bitwise Operators
Now, let us go through each of the operator categories.
1. Arithmetic Operators
Arithmetic Operators are used to perform basic mathematical arithmetic operators like addition, subtraction, multiplication, etc. The following table lists out all the arithmetic operators in Swift.
Operator Symbol | Name | Example | Description |
---|---|---|---|
+ | Addition | x + y | Returns the sum of values in x and y. |
– | Subtraction | x – y | Returns the subtraction y from x. |
* | Multiplication | x * y | Returns the product of values in x and y. |
/ | Division | x / y | Returns the quotient in the division of x by y. |
% | Modulus | x % y | Returns the remainder in the division of x by y. |
In the following program, we will take values for variables x and y, and perform arithmetic operations on these values using Swift Arithmetic Operators.
main.swift
var x = 5
var y = 2
var result = 0
print("Addition")
result = x + y
print(result)
print("\nSubtraction")
result = x - y
print(result)
print("\nMultiplication")
result = x * y
print(result)
print("\nDivision")
result = x / y
print(result)
print("\nModulo")
result = x % y
print(result)
Output
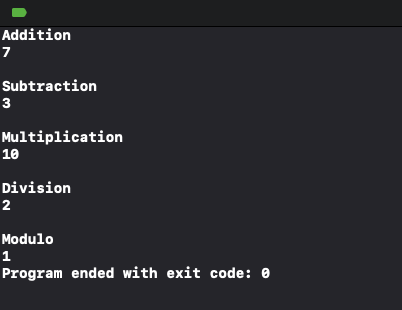
2. Assignment Operators
Assignment Operators are used to assign or store a specific value in a variable. The following table lists out all the assignment operators in Swift.
Operator Symbol | Description | Example | Equivalent to |
---|---|---|---|
= | Assignment | x = y | |
+= | Addition Assignment | x += y | x = x + y |
-= | Subtraction Assignment | x -= y | x = x – y |
*= | Multiplication Assignment | x *= y | x = x * y |
/= | Division Assignment | x /= y | x = x / y |
%= | Modulus Assignment | x %= y | x = x % y |
**= | Exponentiation Assignment | x **= y | x = x ** y |
//= | Floor-division Assignment | x //= y | x = x // y |
&= | AND Assignment | x &= y | x = x & y |
|= | OR Assignment | x |= y | x = x | y |
^= | XOR Assignment | x ^= y | x = x ^ y |
<<= | Zero fill left shift Assignment | x <<= y | x = x << y |
>>= | Signed right shift Assignment | x >>= y | x = x >> y |
In the following program, we will take values for variables x and y, and perform assignment operations on these values using Swift Assignment Operators.
main.py
x, y = 5, 2
x += y
print(x) # 7
x, y = 5, 2
x -= y
print(x) # 3
x, y = 5, 2
x *= y
print(x) # 10
x, y = 5, 2
x /= y
print(x) # 2.5
x, y = 5, 2
x %= y
print(x) # 1
x, y = 5, 2
x **= y
print(x) # 25
x, y = 5, 2
x //= y
print(x) # 2
x, y = 5, 2
x &= y
print(x) # 0
x, y = 5, 2
x |= y
print(x) # 7
x, y = 5, 2
x ^= y
print(x) # 7
x, y = 5, 2
x <<= y
print(x) # 20
x, y = 5, 2
x >>= y
print(x) # 1
3. Bitwise Operators
Bitwise Operators are used to perform bit level operations. The following table lists out all the bitwise operators in Swift.
Operator Symbol | Description | Example |
---|---|---|
& | AND | x & y |
| | OR | x | y |
^ | XOR | x ^ y |
~ | NOT | ~x |
<< | Zero fill left shift | x << y |
>> | Signed right shift | x >> y |
main.py
# AND
x, y = 5, 2
print(x & y) # 0
# OR
x, y = 5, 2
print(x | y) # 7
# XOR
x, y = 5, 2
print(x ^ y) # 7
# NOT
x, y = 5, 2
print(~x) # -6
# Zero fill left shift
x, y = 5, 2
print(x << y) # 20
#Signed right shift
x, y = 5, 2
print(x >> y) # 1
4. Comparison Operators
Comparison Operators are used to compare two operands. The following table lists out all the Comparison operators in Swift.
Operator Symbol | Description | Example |
---|---|---|
== | Equal to | x == y |
!= | Not Equal to | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
main.py
# Equal to
x, y = 5, 2
print(x == y) # False
# Not equal to
x, y = 5, 2
print(x != y) # True
# Greater than
x, y = 5, 2
print(x > y) # True
# Less than
x, y = 5, 2
print(x < y) # False
# Greater than or equal to
x, y = 5, 2
print(x >= y) # True
# Less than or equal to
x, y = 5, 2
print(x <= y) # False
5. Logical Operators
Logical Operators are used to combine simple conditions and form compound conditions. The following table lists out all the Logical operators in Swift.
Operator Symbol | Description | Example |
---|---|---|
and | Returns True if both operands are True. | x and y |
or | Returns True if any of the operands is True. | x or y |
not | Returns the complement of given boolean operand. | not x |
main.py
# Logical AND
x, y = True, False
print(x and y) # False
# Logical OR
x, y = True, False
print(x or y) # True
# Logical NOT
x = True
print(not x) # False
Conclusion
In this Swift Tutorial, we learned about different kinds of Operators in Swift language: Arithmetic, Assignment, Comparison, Logical, and Bitwise, with examples.