Swift Optionals
In Swift, optionals are a powerful feature that allows variables to hold either a value or nil
(no value). They are particularly useful for handling situations where a value may be absent, enabling safer and more predictable code.
What Are Optionals?
An optional is a variable that can either hold a value or be nil
. Optionals are declared by adding a question mark (?
) after the type. For example:
File: main.swift
var optionalName: String? = "Arjun"
print(optionalName) // Optional("Arjun")
optionalName = nil
print(optionalName) // nil
Explanation:
- The variable
optionalName
can hold aString
ornil
. - Initially, it is set to
"Arjun"
, and later, it is set tonil
.
Output:
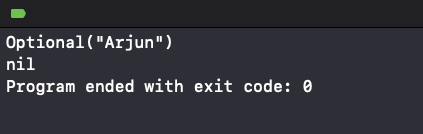
Optional("John Doe")
nil
Unwrapping Optionals
Since optionals may contain nil
, Swift requires them to be unwrapped before accessing their value. Here are some ways to unwrap optionals:
1 Forced Unwrapping
Forced unwrapping uses the exclamation mark (!
) to access the value of an optional:
File: main.swift
var optionalName: String? = "Arjun"
print(optionalName!) // Arjun
Note: Forced unwrapping causes a runtime error if the optional is nil
. Use it only when you are sure the optional contains a value.
Output:
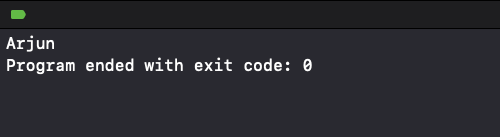
2 Optional Binding
Optional binding safely unwraps an optional by checking if it contains a value:
File: main.swift
var optionalName: String? = "Arjun"
if let name = optionalName {
print("Hello, \(name)!")
} else {
print("No name provided.")
}
Explanation:
- If
optionalName
contains a value, it is unwrapped and assigned toname
. - If
optionalName
isnil
, theelse
block executes.
Output:
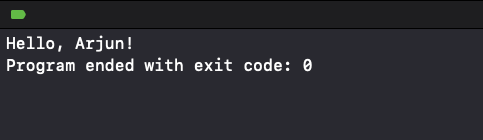
3 Nil Coalescing Operator
The nil coalescing operator (??
) provides a default value if the optional is nil
:
File: main.swift
var optionalName: String? = nil
let name = optionalName ?? "Anonymous"
print("Hello, \(name)!")
Explanation:
- If
optionalName
isnil
, the default value"Anonymous"
is used. - Otherwise, the value of
optionalName
is used.
Output:
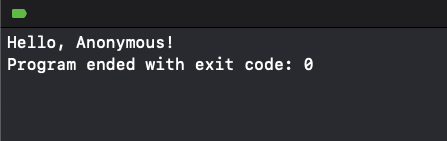
Optional Chaining
Optional chaining allows you to safely access properties, methods, and subscripts of an optional that might be nil
:
File: main.swift
class Person {
var name: String?
}
let person = Person()
person.name = "Arjun"
if let name = person.name?.uppercased() {
print("Uppercased name: \(name)")
} else {
print("No name provided.")
}
Explanation:
- The optional chaining operator (
?.
) safely accesses theuppercased
method ofname
. - If
name
isnil
, the expression evaluates tonil
.
Output:
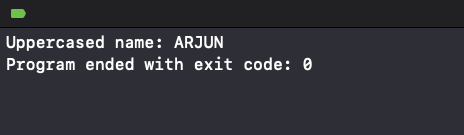