Swift Properties
Properties are variables or constants that are associated with a class, structure, or enumeration in Swift. They define the data that objects of a type can store. Swift provides several types of properties, including stored properties, computed properties, and static properties, which allow developers to model data and behaviors effectively.
Basics of Properties
Properties in Swift are categorized into:
- Stored Properties: Store constant or variable values as part of an instance.
- Computed Properties: Do not store values directly but compute them dynamically.
- Static Properties: Associated with the type itself rather than an instance.
Stored Properties
Stored properties store constant (let
) or variable (var
) values for instances of a class or structure.
Example: main.swift
class Student {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
let student = Student(name: "Arjun", age: 20)
print("Name: \(student.name), Age: \(student.age)")
Explanation:
name
andage
are stored properties of theStudent
class.- These properties are initialized via the initializer and accessed using the dot operator.
Output:
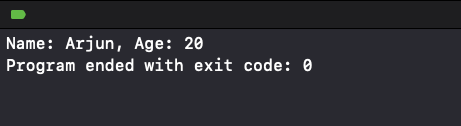
Computed Properties
Computed properties compute their value dynamically rather than storing it directly. They use get
and optionally set
blocks to define their behavior.
Example: main.swift
class Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
var area: Double {
return width * height
}
}
let rect = Rectangle(width: 5.0, height: 10.0)
print("Area: \(rect.area)")
Explanation:
area
is a computed property that calculates the rectangle’s area usingwidth
andheight
.- It does not store the value but computes it each time it is accessed.
Output:
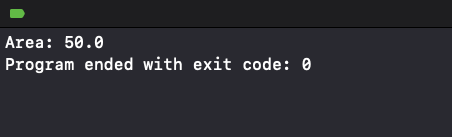
Getters and Setters for Computed Properties
Computed properties can have a set
block to allow modification of their values.
Example: main.swift
class Rectangle {
var width: Double
var height: Double
init(width: Double, height: Double) {
self.width = width
self.height = height
}
var area: Double {
get {
return width * height
}
set {
width = newValue / height
}
}
}
let rect = Rectangle(width: 5.0, height: 10.0)
print("Original Area: \(rect.area)")
rect.area = 100.0
print("New Width: \(rect.width)")
Explanation:
- The
get
block calculates the area of the rectangle. - The
set
block adjusts the width based on the new area value. newValue
is a default parameter available in theset
block.
Output:
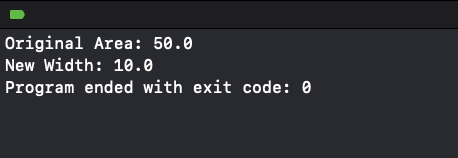
Static Properties
Static properties belong to the type itself rather than any specific instance. They are defined using the static
keyword.
Example: main.swift
class Circle {
static let pi = 3.14159
var radius: Double
init(radius: Double) {
self.radius = radius
}
var circumference: Double {
return 2 * Circle.pi * radius
}
}
let circle = Circle(radius: 5.0)
print("Circumference: \(circle.circumference)")
Explanation:
pi
is a static property shared by all instances of theCircle
class.- It is accessed using the type name (
Circle.pi
). - The
circumference
property usespi
to calculate the circumference of the circle.
Output:
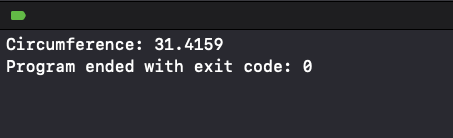