Swift – Sort String Array based on String Length
To sort a String Array based on string length, call sort(by:)
method on the string array, and give the predicate to by
parameter of sort(by:)
method, such that the sorting happens based on string length.
If the original array has to be preserved use sorted(by:)
method.
Example
In the following program, we will take an array of strings, and sort them based on length using sort()
method.
main.swift
var ids = ["ab", "qprs", "mno", "vwxyz", "c"]
ids.sort(by: {$0.count < $1.count})
print(ids)
Output
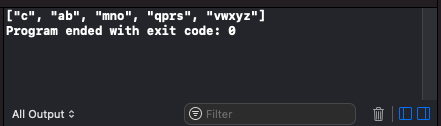
Let us try the same scenario with sorted(by:)
method.
main.swift
var strArr = ["ab", "qprs", "mno", "vwxyz", "c"]
var sortedArr = strArr.sorted(by: {$0.count < $1.count})
print("Original Array: \(strArr)")
print("Sorted Array: \(sortedArr)")
Output
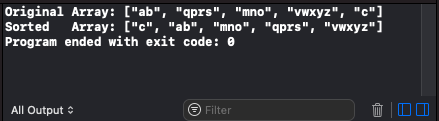
The given predicate in the above programs makes the sorting happen in ascending order. To sort in descending order, change the predicate to as shown in the following program.
main.swift
var ids = ["ab", "qprs", "mno", "vwxyz", "c"]
ids.sort(by: {$0.count > $1.count})
print(ids)
Output
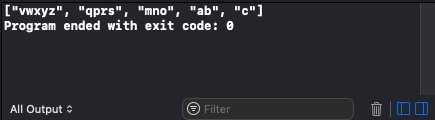
Conclusion
In this Swift Tutorial, we learned how to sort the strings in an Array based on their length using sort(by:) or sorted(by:) method.