Introduction to Strings in Swift
Strings are a fundamental data type in Swift used to represent text. A string is a sequence of characters, such as words, sentences, or even empty text. Swift provides powerful tools for creating, modifying, and manipulating strings, making them highly versatile.
Creating Strings
Strings can be created using string literals enclosed in double quotes:
File: main.swift
let greeting: String = "Hello, Swift!"
let emptyString = "" // An empty string
print(greeting)
print("Is the string empty? \(emptyString.isEmpty)")
Output:
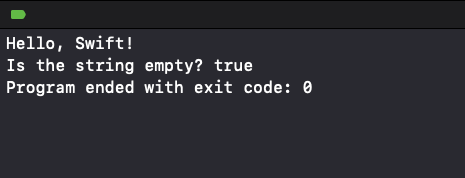
String Interpolation
String interpolation allows you to include variables or expressions inside a string:
File: main.swift
let name = "Arjun"
let age = 25
let message = "My name is \(name) and I am \(age) years old."
print(message)
Explanation:
- The variables
name
andage
are embedded into the string using\()
.
Output:
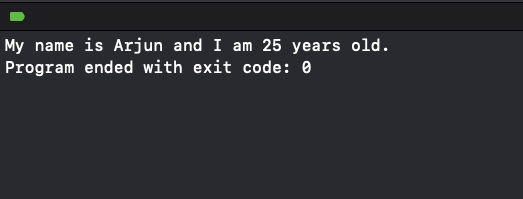
String Concatenation
Strings can be combined using the +
operator:
File: main.swift
let part1 = "Hello, "
let part2 = "World!"
let combined = part1 + part2
print(combined)
Output:
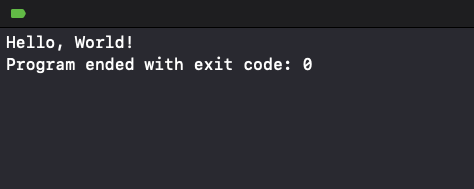
String Properties and Methods
Strings in Swift have several useful properties and methods:
File: main.swift
let text = "Swift Programming"
print("Length of the string: \(text.count)")
print("Does it start with 'Swift'? \(text.hasPrefix("Swift"))")
print("Does it end with 'Programming'? \(text.hasSuffix("Programming"))")
Output:
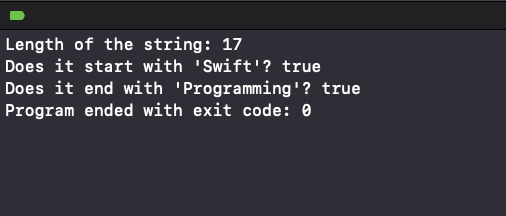
Iterating Over Characters
You can iterate over the characters in a string:
File: main.swift
let word = "Swift"
for char in word {
print(char)
}
Output:
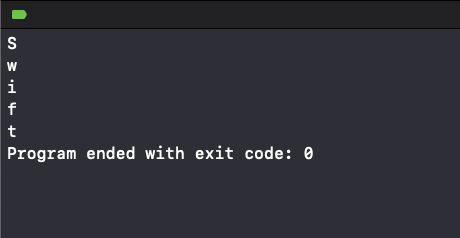
Multi-line Strings
Swift supports multi-line strings using triple double quotes:
File: main.swift
let poem = """
Roses are red,
Violets are blue,
Swift is awesome,
And so are you!
"""
print(poem)
Output:
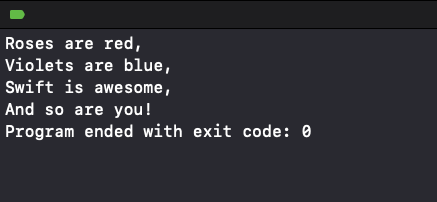