Swift Type Aliases
In Swift, type aliases provide a way to give an alternative name to an existing type. This feature is particularly useful for making code more readable and meaningful, especially when dealing with complex types.
What Are Type Aliases?
A type alias is a new name for an existing type. You create a type alias using the typealias
keyword. Type aliases do not create new types; they simply provide a more descriptive name for existing types.
Syntax:
typealias NewName = ExistingType
Using Type Aliases
Here’s an example of creating and using a type alias:
File: main.swift
typealias Age = Int
let myAge: Age = 25
print("My age is \(myAge)")
Explanation:
Age
is defined as a type alias forInt
.- The variable
myAge
is declared asAge
, which is equivalent toInt
.
Output:
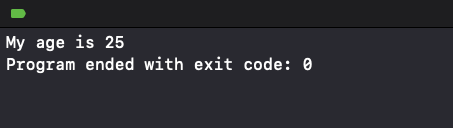
Type Aliases for Complex Types
Type aliases are particularly useful for simplifying complex types:
File: main.swift
typealias Coordinate = (x: Double, y: Double)
let point: Coordinate = (x: 10.5, y: 20.0)
print("Point is at (\(point.x), \(point.y))")
Explanation:
Coordinate
is a type alias for a tuple withx
andy
asDouble
.- The variable
point
is declared as aCoordinate
, simplifying the tuple type.
Output:
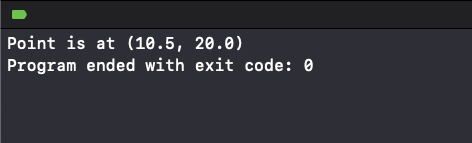
Type Aliases for Function Types
You can use type aliases to simplify function types:
File: main.swift
typealias Operation = (Int, Int) -> Int
let add: Operation = { (a, b) in a + b }
let result = add(5, 3)
print("Result: \(result)")
Explanation:
Operation
is a type alias for a function type that takes twoInt
parameters and returns anInt
.- The variable
add
is a closure that matches theOperation
type.
Output:
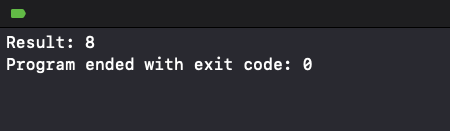
Type Aliases with Protocols
Type aliases can be used to simplify protocol conformance:
File: main.swift
protocol Drawable {
func draw()
}
typealias Shape = Drawable
struct Circle: Shape {
func draw() {
print("Drawing a circle")
}
}
let circle = Circle()
circle.draw()
Explanation:
Shape
is a type alias for theDrawable
protocol.- The
Circle
struct conforms to theShape
(orDrawable
) protocol.
Output:
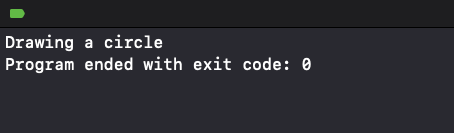
Conclusion
Type aliases in Swift improve code readability and maintainability by providing meaningful names for existing types. They are especially useful for simplifying complex or repetitive types.