What are Variables in Swift?
In Swift, variables are used to store data that can change during the execution of a program. Variables allow you to label and manipulate data easily, making your code more readable and maintainable.
In this tutorial, you will learn how to declare variables, and how to use them in Swift programs to read values from variables, update values in variables, with detailed examples.
Declaring Variables
To declare a variable in Swift, use the var
keyword followed by the variable name and optional type annotation. For example:
var variableName: DataType = value
If the type of the variable can be inferred from the value, the type annotation is optional.
Example 1: Declaring and Using Variables
In this example, we will declare and use variables of different data types like integer, string, and boolean, and read the values in variable, and print them to output.
File: main.swift
var age: Int = 25
var name: String = "Alice"
var isStudent: Bool = true
print("Name: \(name)")
print("Age: \(age)")
print("Is Student: \(isStudent)")
Explanation:
age
is declared as an integer variable and assigned the value25
.name
is declared as a string variable and assigned the value"Alice"
.isStudent
is declared as a boolean variable and assigned the valuetrue
.- The
print
statements output the values of the variables using string interpolation (e.g.,\(variableName)
).
Output:
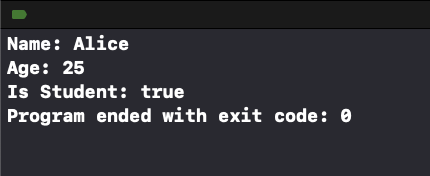
Example 2: Updating Variable Values
In this example, we will initialise a variable and then update the variable by reassigning with another value, and print the updated value to output.
File: main.swift
var score = 10
print("Initial score: \(score)")
// Updating the value of score
score = 20
print("Updated score: \(score)")
Explanation:
score
is declared and initialized with the value10
.- The value of
score
is updated to20
. - The updated value is printed, showing that the variable can change its value during execution.
Output:
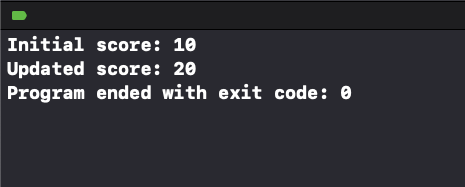
Example 3: Combining Variables and Constants
In Swift, if you want a value to remain constant (unchangeable), use the let
keyword instead of var
.
In the following program, we will demonstrate how to use constants and variables together.
File: main.swift
let base = 10
var height = 5
let area = base * height
print("Area of the rectangle: \(area)")
Explanation:
base
is declared as a constant with a value of10
.height
is declared as a variable with a value of5
.- The
area
is calculated by multiplyingbase
andheight
. Sincebase
is constant andheight
can change, this setup provides flexibility while preserving immutability forbase
.
Output:
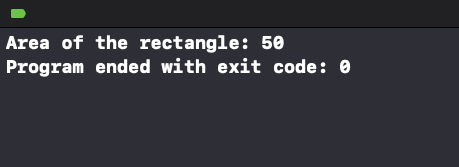
Type Inference in Swift
Swift can often infer the type of a variable or constant from its initial value, so you can omit the type annotation:
File: main.swift
var message = "Hello, Swift!" // Swift infers that message is of type String
var number = 42 // Swift infers that number is of type Int
print("Message: \(message)")
print("Number: \(number)")
Output:
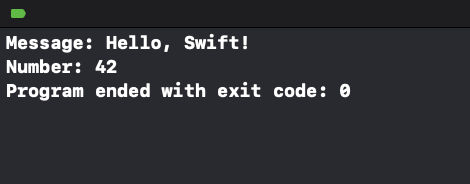
Conclusion
Variables and constants are fundamental concepts in Swift. Use var
for values that can change and let
for values that should remain constant.