Controller extension in Salesforce is an Apex class containing a constructor that is used to add or extend the functionalities of a Standard Controller or custom controller in Salesforce. Extension controller is also used to leverage the functionality of another controller using our own custom logic. Controller extensions in salesforce can be used without Standard controller or custom controller.
Controller extension in Salesforce
In this Salesforce Tutorial, we are going to learn about Controller extension in Salesforce and how can we build Controller extension in Salesforce. Let us learn about controller extension with a simple example.
- Navigate to Setup | Build | Develop | Apex classes | New.
In this Salesforce Visualforce tutorial, we are going to build Salesforce extension controller by creating Apex class. A controller extension is any Apex class containing a constructor that takes a single argument of type ApexPages.StandardController or CustomControllerName, where ExtensionController is the name of a custom controller in this example.
public class ExtensionController {
private final Account acct;
public ExtensionController {
(ApexPages.StandardController stdController) {
this.acct = (Account)stdController.getRecord();
}
public String getGreeting() {
return 'Hello ' + acct.name + ' (' + acct.id + ')';
}
}
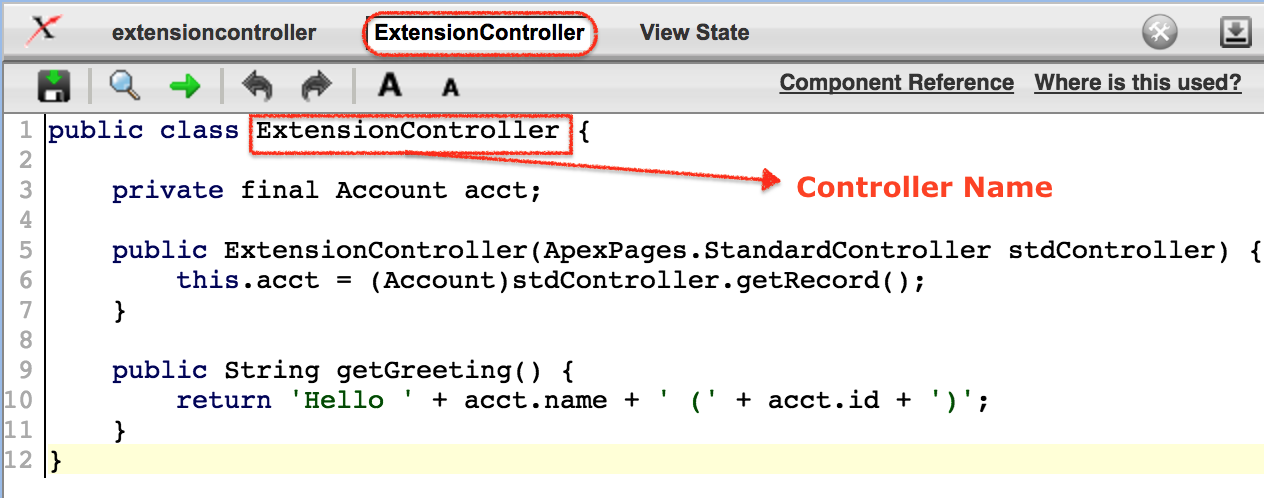
- {!greeting} expression references the getGreeting method in extension controller.
After creating Controller extension in Salesforce by creating an Apex class, now we have to implement Controller extension in Salesforce using visualforce pages. Let us create visualforce page as shown below.
- In this example, we have named the Visualforce page as extensioncontroller. The extension controller is added to visualforce page using the extension attribute in <apex:page> component. Both standard controller and extension attributes must be used in <apex:page> component.
<apex:page standardController="Account" extensions="ExtensionController">
{!greeting} <p/>
<apex:form >
<apex:inputField value="{!account.name}"/> <p/>
<apex:commandButton value="Save" action="{!save}"/>
</apex:form>
</apex:page>
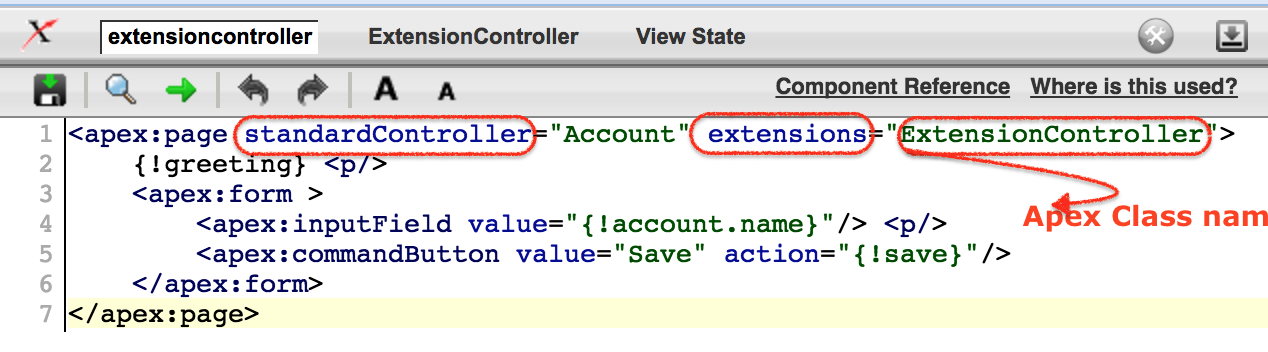
Every controller methods and controller extension methods are referenced with {!} notation in visualforce markup.
- <apex:inputField> tag retrieves the name of the account using standard controller.
- <apex:commandButton> tag saves the method with its action attribute.
Visualforce extension controller Output.
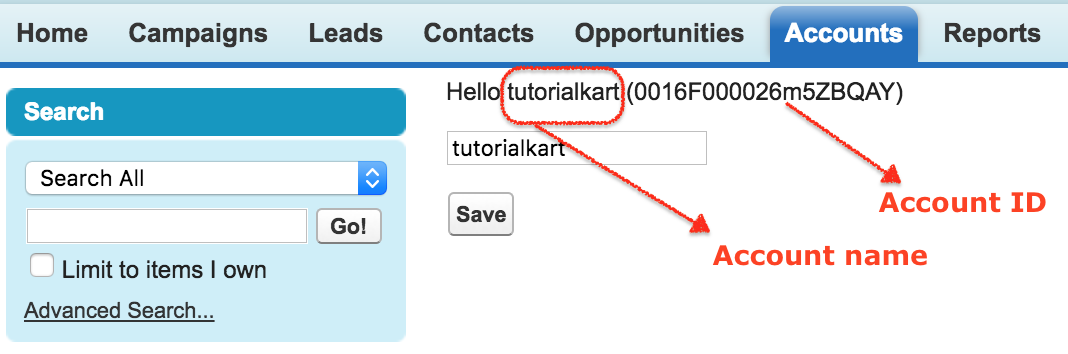
The above shown image is the output for extension controller in visualforce. Account Id must be added at the end of the URl and click on Save button to get the output. As shown above the the name of the account is tutorialkart, if we change the name and Saved. The account named is renamed as shown below.
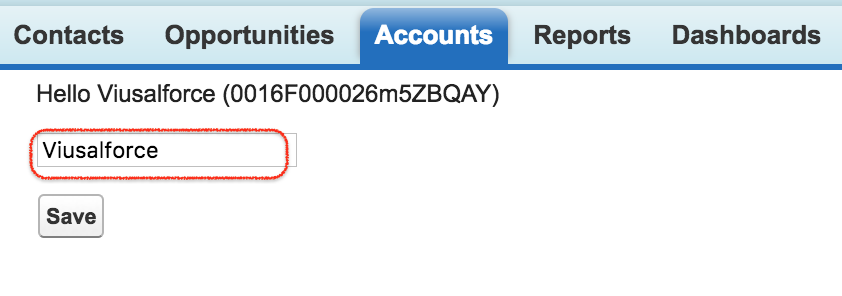
Important points that to be remembered before creating custom and extension controllers in salesforce.
- Governor limits.
- Apex class can be run in the system mode and user mode by using without sharing and with sharing.
- The webservice method must be defined as global.
- We can not implement DML (Data manipulation Language) constructor method of a controller.
- try to use Sets, Maps and List in your code, it increases the efficiency of the code.