Flutter Animate Color
In this tutorial, we will learn how to animate color of a widget, i.e., transitioning from a starting color to ending color.
You can animate color of a widget using ColorTween.
In the following example, we shall animate color of a button, when it is pressed.
main.dart
import 'package:flutter/animation.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> with SingleTickerProviderStateMixin {
Animation<Color> animation;
AnimationController controller;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 1), vsync: this);
animation =
ColorTween(begin: Colors.indigo, end: Colors.lime).animate(controller)
..addListener(() {
setState(() {
// The state that has changed here is the animation object’s value.
});
});
}
void animateColor() {
controller.forward();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Center(child: Text('Flutter - tutorialkart.com')),
),
body: ListView(children: <Widget>[
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.all(20),
height: 400,
child: RaisedButton(
onPressed: () => {animateColor()},
color: animation.value,
child: Text(''),
))
]),
));
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
}
When you run this application, and click on the big square button which is colored indigo, animation of color starts and changes the color of button to limegreen.
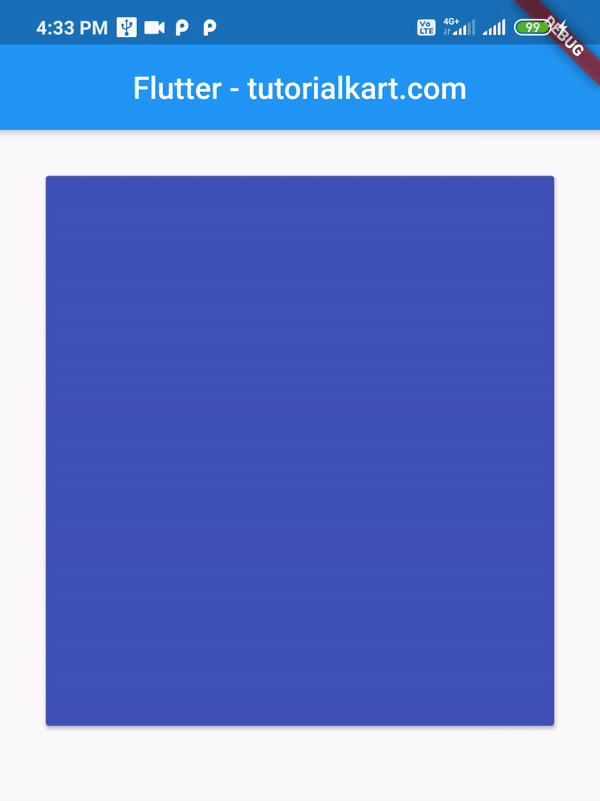
Now let us modify the application, such that the color toggles when button is pressed.
We shall use controller.reverse() function to reverse the animation.
main.dart
import 'package:flutter/animation.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> with SingleTickerProviderStateMixin {
Animation<Color> animation;
AnimationController controller;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 1), vsync: this);
animation =
ColorTween(begin: Colors.indigo, end: Colors.lime).animate(controller)
..addListener(() {
setState(() {
// The state that has changed here is the animation object’s value.
});
});
}
bool buttonToggle = true;
void animateColor() {
if (buttonToggle) {
controller.forward();
} else {
controller.reverse();
}
buttonToggle = !buttonToggle;
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Center(child: Text('Flutter - tutorialkart.com')),
),
body: ListView(children: <Widget>[
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.all(20),
height: 400,
child: RaisedButton(
onPressed: () => {animateColor()},
color: animation.value,
child: Text(''),
))
]),
));
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
}
When you run this application and click on the colored button, color animation toggles using controller.forward() and controller.reverse().
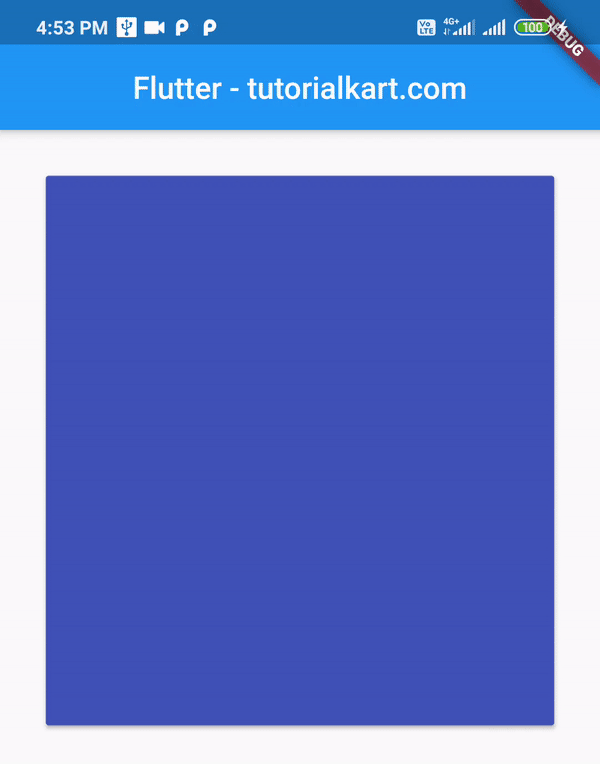
Conclusion
In this Flutter Tutorial, we learned how to animate Color property using Animation and AnimationController classes.