Flutter – Container Background Color
To set background color for Container widget, set its color
property with the required Color value or set the decoration
property with required background color value in it.
Sample Code
A quick code snippet to set the background color for a Container widget using color
property is
Container( color: Colors.green, )
A quick code snippet to set the background color for a Container widget using decoration
property is
Container( decoration: const BoxDecoration( borderRadius: BorderRadius.all(Radius.circular(15)), ), )
Examples
Container Background Color using color Property
In the following example, we create a Flutter Application with a Container widget, and set its background color to green using color
property of this Container widget.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return Center( child: Column( children: <Widget>[ const SizedBox(height: 10,), Container( height: 150, width: 200, color: Colors.green[200], alignment: Alignment.center, child: const Text('Hello World'), ), ], ), ); } }
Container Background Color using decoration Property
In the following example, we create a Flutter Application with a Container widget, and set its background color to green using decoration
property of this Container widget.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return Center( child: Column( children: <Widget>[ const SizedBox(height: 10,), Container( height: 150, width: 200, decoration: BoxDecoration( color: Colors.green[200], ), alignment: Alignment.center, child: const Text('Hello World'), ), ], ), ); } }
Screenshot – iPhone Simulator
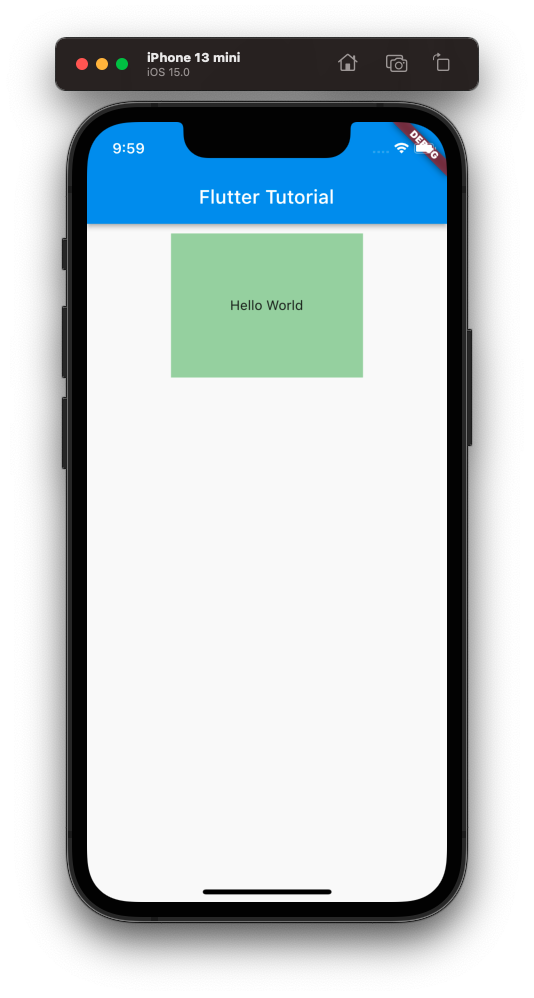
Screenshot – Android Emulator
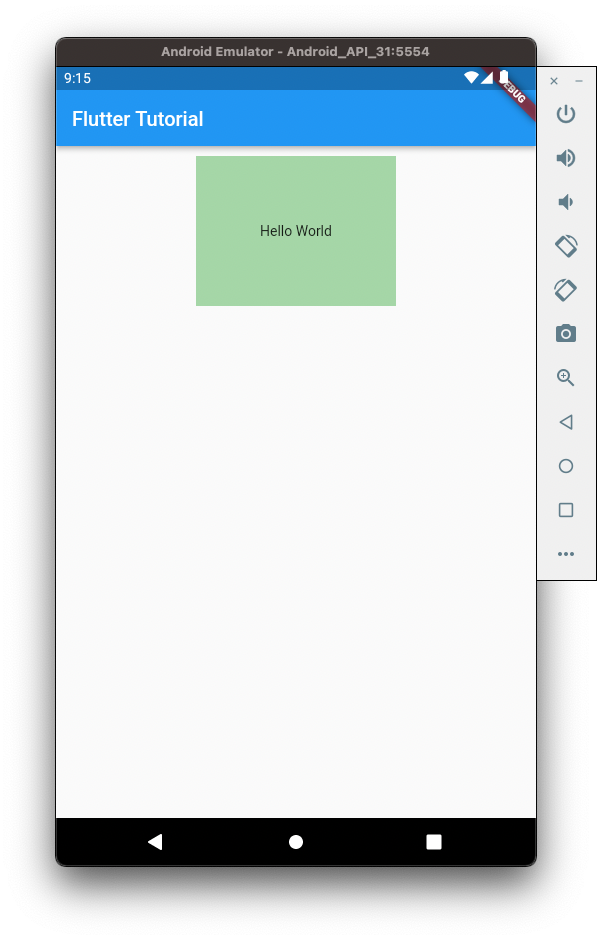
Conclusion
In this Flutter Tutorial, we learned how to set background color for Container widget, with examples.