Flutter Round Image
Flutter Image widget is used to display an image in your Android or IOS application. Usually, the image is of rectangular shape. But, if you would like to display it with a circular shape, you can do so using BoxDecoration and DecorationImage widgets.
Use a Container that has BoxDecoration with the circle shape and DecorationImage that shows our image. As a result, we shall get a round image, or a an image with the circular shape defined by the Container’s decoration.
Example – Flutter Round Image
In this example Flutter Application, we shall display an image using Image widget. And also another widget that shows the image in a Circular shape. The first one is just for comparison of how we display an image, that is rectangular, in a round shape.
By using a Container with BoxDecoration whose shape is circular and uses the image as DecoratioImage, we can shown the rectangular image as a circular shaped image.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Image - tutorialkart.com'),
),
body: Center(
child: Container(
color: Colors.cyan,
width: double.infinity,
child: Column(children: <Widget>[
Container(
height: 150,
width: 150,
margin: EdgeInsets.all(10),
child: Image(
image: NetworkImage(
'https://www.tutorialkart.com/img/hummingbird.png'),
),
),
Container(
margin: EdgeInsets.all(10),
width: 150,
height: 150,
decoration: BoxDecoration(
shape: BoxShape.circle,
image: DecorationImage(
image: NetworkImage(
'https://www.tutorialkart.com/img/hummingbird.png'
),
fit: BoxFit.fill
),
),
),
]),
),
),
),
);
}
}
Run the application on an Android Emulator or connect a Physical Device to your computer and try running on this device. The widgets shall appear on the screen as shown in the below screenshot.
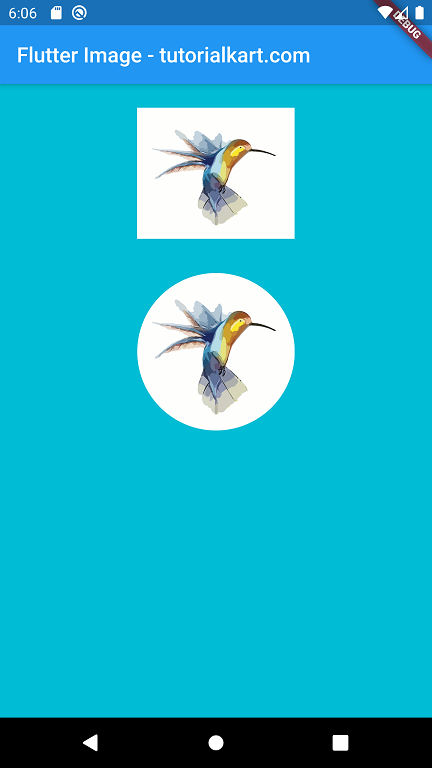
The first widget that displays the image is only for comparison purpose. The second widget is the actual circular or round image which we are trying to demonstrate in this tutorial.
Conclusion
In this Flutter Tutorial, we learned how to display an image in the shape of a circular disc.