Flutter Padding
Flutter Padding – You can provide padding to some of the widgets in Flutter. In this tutorial, we will focus on giving padding to a Container widget. But the syntax should be same for any widget that supports padding property.
You can provide padding to a widget in many ways. Following are some of them.
- EdgeInsets.all()
- EdgeInsets.fromLTRB()
- EdgeInsets.only()
The quick code snippets for these functions is provided below.
///same padding to all the four sides: left, top, right, bottom
padding: EdgeInsets.all(10)
///provide padding to left, top, right, bottom respectively
padding: EdgeInsets.fromLTRB(10, 15, 20, 5)
///provide padding only to the specified sides
padding: EdgeInsets.only(left: 20, top:30)
Example – Padding to Container Widget
In this example, we provide padding to a Container widget in some of the different possible scenarios.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter - tutorialkart.com'),
),
body: Center(child: Column(children: <Widget>[
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.all(5),
color: Colors.green,
child: Container(
width: 150,
height: 50,
color: Colors.white70,
child: Text(''),
),
),
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.fromLTRB(20, 30, 10, 15),
color: Colors.green,
child: Container(
width: 150,
height: 50,
color: Colors.white70,
child: Text(''),
),
),
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.only(left: 50),
color: Colors.green,
child: Container(
width: 150,
height: 50,
color: Colors.white70,
child: Text(''),
),
),
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.only(left: 20, right: 10),
color: Colors.green,
child: Container(
width: 150,
height: 50,
color: Colors.white70,
child: Text(''),
),
),
Container(
margin: EdgeInsets.all(10),
padding: EdgeInsets.only(top: 20, bottom: 10),
color: Colors.green,
child: Container(
width: 150,
height: 50,
color: Colors.white70,
child: Text(''),
),
),
]))),
);
}
}
Output
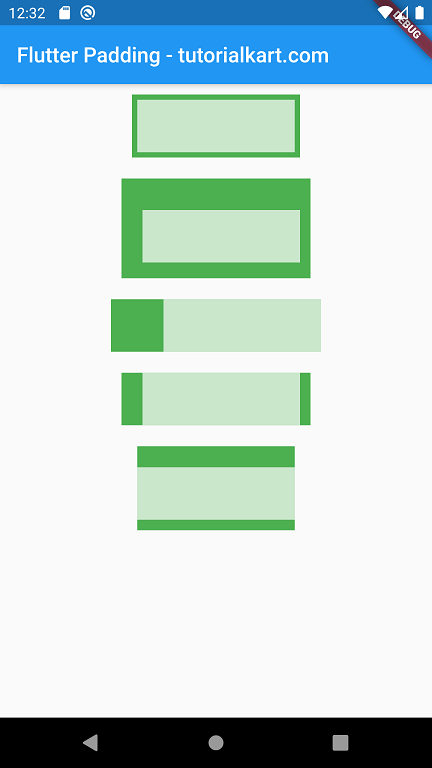
Complete Code in GitHub
You can get the complete code of the Flutter Application used in the above examples at the following link.
https://github.com/tutorialkart/flutter/tree/master/flutter_padding_tutorial.
Conclusion
In this Flutter Tutorial, we learned about providing padding to a widget and its usage with examples for different scenarios.