Flutter ToggleButtons
In this tutorial, we will learn how to use ToggleButtons widget.
Following is a simple and quick code snippet on how to use ToggleButtons widget. Following code should go to your State class.
</>
Copy
List<bool> _selections = List.generate(3, (_)=> false);
@override
Widget build(BuildContext context) {
...
ToggleButtons(
children: <Widget>[
Icon(Icons.add_comment),
Icon(Icons.airline_seat_individual_suite),
Icon(Icons.add_location),
],
isSelected: _selections,
onPressed: (int index) {
setState(() {
_selections[index] = !_selections[index];
});
},
)
...
}
Example
In the following program, we use ToggleButtons widget with three icon widgets as children.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
List<bool> _selections = List.generate(3, (_) => false);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Center(child: Text('Flutter - tutorialkart.com')),
),
body: ListView(children: <Widget>[
Container(
alignment: Alignment.center,
margin: EdgeInsets.all(10),
padding: EdgeInsets.all(20),
child: ToggleButtons(
children: <Widget>[
Icon(Icons.add_comment),
Icon(Icons.airline_seat_individual_suite),
Icon(Icons.add_location),
],
isSelected: _selections,
onPressed: (int index) {
setState(() {
_selections[index] = !_selections[index];
});
},
))
]),
));
}
}
When you run this application, you will get UI as shown below.
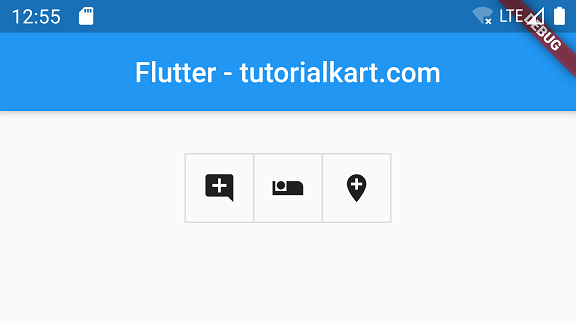
When you click on a toggle button, the color and background color changes as shown below.
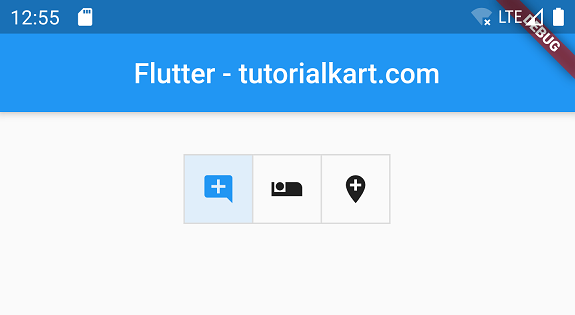
If you click on the same button again, the it comes to its original state. Also you can press on multiple toggle buttons, although state of each button is stored separately.
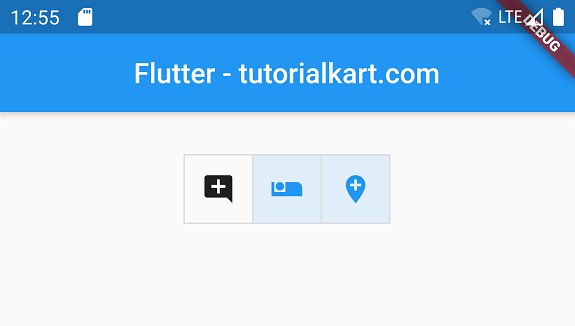
Conclusion
In this Flutter Tutorial, we learned how to use ToggleButtons.