In this tutorial, you shall learn how to copy a directory (all the files and sub-directories in it) to a new path in Kotlin using copyRecursively() function, with example programs.
Kotlin – Copy directory
To copy a directory in Kotlin, where you copy all the files and sub-directories, you can use copyRecursively() function of java.io.File class.
Steps to copy a directory
- Consider that we would like to copy a directory from source location to target location.
- Create two file objects: one with the source directory path, and the other with target directory path, using java.io.File class.
- Call copyRecursively() function on the source directory object, and pass the target directory object as argument. The function copies the source directory to target directory.
val sourceDir = File("source/directory") val targetDir = File("target/directory") sourceDir.copyRecursively(targetDir)
ADVERTISEMENT
Example
For this example, we will consider a directory at the path mydata/set1/
.
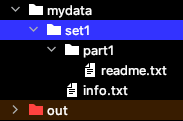
In the following program, we copy the directory mydata/set1/
to mydata/set2/
.
Main.kt
import java.io.File import java.lang.Exception fun main() { val sourceDir = File("mydata/set1/") val targetDir = File("mydata/set2/") try { if (sourceDir.copyRecursively(targetDir)) { println("Directory copied successfully.") } else { println("Directory not copied successfully.") } } catch (e: Exception) { println("Exception occurred while copying.") } }
Output
Directory copied successfully.
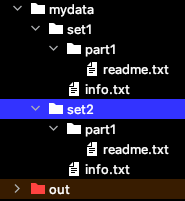
Conclusion
In this Kotlin Tutorial, we learned how to copy a directory recursively using File.copyRecursively() function.